1000
|
It is possible to search for an item ( inside the Editor ), case insensitive
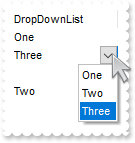
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IEditorPtr var_Editor = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"DropDownList")))->GetEditor();
var_Editor->PutEditType(EXGRIDLib::DropDownListType);
var_Editor->AddItem(1,L"One",vtMissing);
var_Editor->AddItem(2,L"Two",vtMissing);
var_Editor->AddItem(3,L"Three",vtMissing);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem(spGrid1->GetColumns()->GetItem(long(0))->GetEditor()->GetFindItem(">ONE"));
var_Items->AddItem(spGrid1->GetColumns()->GetItem(long(0))->GetEditor()->GetFindItem(">ThRee"));
var_Items->AddItem(spGrid1->GetColumns()->GetItem(long(0))->GetEditor()->GetFindItem("ONE"));
var_Items->AddItem(spGrid1->GetColumns()->GetItem(long(0))->GetEditor()->GetFindItem(">tWo"));
spGrid1->EndUpdate();
|
999
|
The text after the BR-tag is in same line as the text before the BR-tag (entire column)
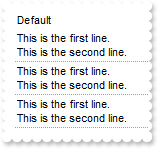
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutDrawGridLines(EXGRIDLib::exHLines);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Default")));
var_Column->PutDef(EXGRIDLib::exCellValueFormat,long(1));
var_Column->PutDef(EXGRIDLib::exCellSingleLine,VARIANT_FALSE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("This is the first line.<br>This is the second line.");
var_Items->AddItem("This is the first line.<br>This is the second line.");
var_Items->AddItem("This is the first line.<br>This is the second line.");
spGrid1->EndUpdate();
|
998
|
The text after the BR-tag is in same line as the text before the BR-tag (individual)
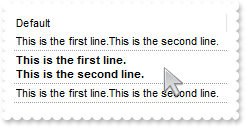
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutDrawGridLines(EXGRIDLib::exHLines);
spGrid1->GetColumns()->Add(L"Default");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValueFormat(var_Items->AddItem("This is the first line.<br>This is the second line."),long(0),EXGRIDLib::exHTML);
long h = var_Items->AddItem("<b>This is the first line.<br>This is the second line.</b>");
var_Items->PutCellValueFormat(h,long(0),EXGRIDLib::exHTML);
var_Items->PutCellSingleLine(h,long(0),EXGRIDLib::exCaptionWordWrap);
var_Items->PutCellValueFormat(var_Items->AddItem("This is the first line.<br>This is the second line."),long(0),EXGRIDLib::exHTML);
spGrid1->EndUpdate();
|
997
|
Can I disable an item once the user selects a new value into a different item
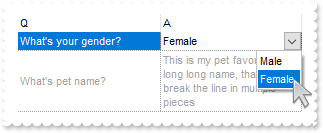
// Change event - Occurs when the user changes the cell's content.
void OnChangeGrid1(long Item,long ColIndex,VARIANT FAR* NewValue)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutEnableItem(var_Items->GetItemByIndex(1),VARIANT_FALSE);
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->FreezeEvents(VARIANT_TRUE);
spGrid1->BeginUpdate();
spGrid1->PutScrollBySingleLine(VARIANT_TRUE);
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
spGrid1->GetColumns()->Add(L"Q");
spGrid1->GetColumns()->Add(L"A");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h1 = var_Items->AddItem("What's your gender?");
EXGRIDLib::IEditorPtr var_Editor = var_Items->GetCellEditor(h1,long(1));
var_Editor->PutEditType(EXGRIDLib::DropDownListType);
var_Editor->AddItem(1,L"Male",vtMissing);
var_Editor->AddItem(0,L"Female",vtMissing);
var_Items->PutCellValue(h1,long(1),long(1));
long h2 = var_Items->AddItem("What's pet name?");
var_Items->PutCellValue(h2,long(1),"This is my pet favorite long long long name, that shoul break the line in multiple pieces");
var_Items->PutCellSingleLine(h2,long(1),EXGRIDLib::exCaptionWordWrap);
spGrid1->EndUpdate();
spGrid1->FreezeEvents(VARIANT_FALSE);
|
996
|
How can I get a row expanded / enlarged to fit the cell's text (entire column)
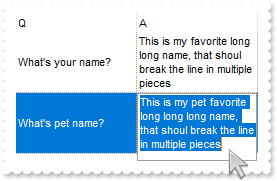
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutScrollBySingleLine(VARIANT_TRUE);
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
spGrid1->GetColumns()->Add(L"Q");
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"A")));
var_Column->PutDef(EXGRIDLib::exCellSingleLine,VARIANT_FALSE);
EXGRIDLib::IEditorPtr var_Editor = var_Column->GetEditor();
var_Editor->PutEditType(EXGRIDLib::MemoType);
var_Editor->PutAppearance(EXGRIDLib::SingleApp);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h1 = var_Items->AddItem("What's name?");
var_Items->PutCellValue(h1,long(1),"This is my pet favorite long long long name, that shoul break the line in multiple pieces");
long h2 = var_Items->AddItem("What's your pet name?");
var_Items->PutCellValue(h2,long(1),"This is my pet favorite long long long name, that shoul break the line in multiple pieces");
spGrid1->EndUpdate();
|
995
|
How can I get a row expanded / enlarged to fit the cell's text (individual cell)
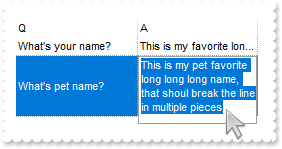
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutScrollBySingleLine(VARIANT_TRUE);
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
spGrid1->GetColumns()->Add(L"Q");
spGrid1->GetColumns()->Add(L"A");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h1 = var_Items->AddItem("What's name?");
var_Items->PutCellValue(h1,long(1),"This is my pet favorite long long long name, that shoul break the line in multiple pieces");
long h2 = var_Items->AddItem("What's your pet name?");
EXGRIDLib::IEditorPtr var_Editor = var_Items->GetCellEditor(h2,long(1));
var_Editor->PutEditType(EXGRIDLib::MemoType);
var_Editor->PutAppearance(EXGRIDLib::SingleApp);
var_Items->PutCellValue(h2,long(1),"This is my pet favorite long long long name, that shoul break the line in multiple pieces");
var_Items->PutCellSingleLine(h2,long(1),EXGRIDLib::exCaptionWordWrap);
spGrid1->EndUpdate();
|
994
|
InsertControlItem / UserEditor / A2X:
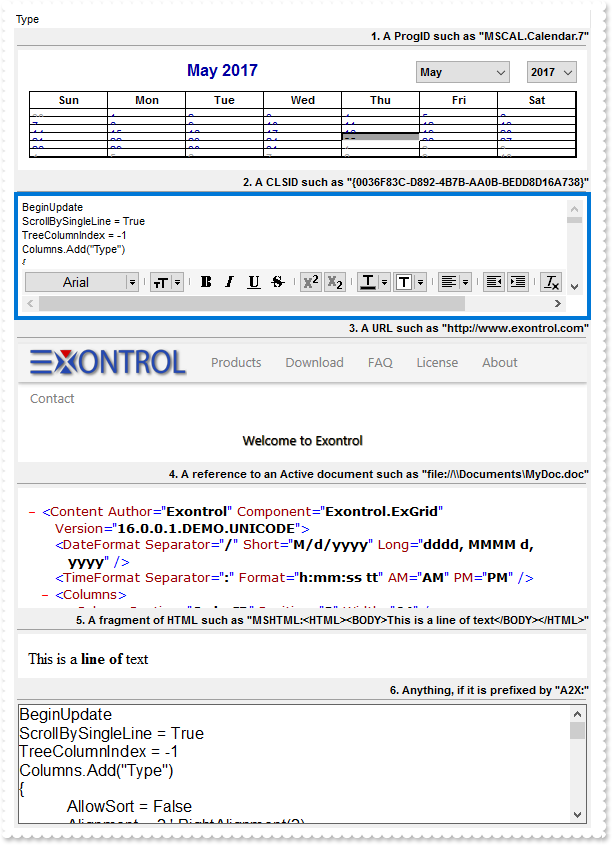
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutBackColor(RGB(240,240,240));
spGrid1->GetConditionalFormats()->Add(L"1 = 1",vtMissing)->PutBold(VARIANT_TRUE);
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Type")))->PutAlignment(EXGRIDLib::RightAlignment);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("1. A ProgID such as \"MSCAL.Calendar.7\"");
var_Items->PutItemDivider(h,0);
long hX = var_Items->InsertControlItem(0,L"MSCAL.Calendar","");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'MSACAL' for the library: 'Microsoft Calendar Control 2007'
#import <MSCAL.OCX>
*/
((MSACAL::ICalendarPtr)(var_Items->GetItemObject(hX)))->PutBackColor(RGB(255,255,255));
h = var_Items->AddItem("2. A CLSID such as \"{0036F83C-D892-4B7B-AA0B-BEDD8D16A738}\"");
var_Items->PutItemDivider(h,0);
hX = var_Items->InsertControlItem(0,L"{0036F83C-D892-4B7B-AA0B-BEDD8D16A738}","");
h = var_Items->AddItem("3. A URL such as \"http://www.exontrol.com\"");
var_Items->PutItemDivider(h,0);
hX = var_Items->InsertControlItem(0,L"http://www.exontrol.com","");
h = var_Items->AddItem("4. A reference to an Active document such as \"file://\\\\Documents\\MyDoc.doc\"");
var_Items->PutItemDivider(h,0);
hX = var_Items->InsertControlItem(0,L"file://C:\\empesting.xml","");
h = var_Items->AddItem("5.A fragment of HTML such as \"MSHTML:<HTML><BODY>This is a line of text</BODY></HTML>\"");
var_Items->PutItemDivider(h,0);
hX = var_Items->InsertControlItem(0,L"MSHTML:<HTML><BODY>This is a <b>line of</b> text</BODY></HTML>","");
var_Items->PutItemHeight(hX,56);
h = var_Items->AddItem("6.Anything, if it is preffixed by \"A2X:\"");
var_Items->PutItemDivider(h,0);
hX = var_Items->InsertControlItem(0,L"A2X:TOC24.Toc24Ctrl.1","");
spGrid1->EndUpdate();
|
993
|
How do I add a RichTextBox editor
// UserEditorOleEvent event - Occurs when an user editor fires an event.
void OnUserEditorOleEventGrid1(LPDISPATCH Object,LPDISPATCH Ev,BOOL FAR* CloseEditor,long Item,long ColIndex)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
OutputDebugStringW( L"Ev" );
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutDrawGridLines(EXGRIDLib::exAllLines);
spGrid1->PutDefaultItemHeight(32);
EXGRIDLib::IEditorPtr var_Editor = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"RICHTEXT")))->GetEditor();
var_Editor->PutEditType(EXGRIDLib::UserEditorType);
var_Editor->UserEditor(L"RICHTEXT.RichtextCtrl",L"");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'RichTextLib' for the library: 'Microsoft Rich Textbox Control 6.0 (SP4)'
#import <richtx32.ocx>
*/
RichTextLib::IRichTextPtr var_RichTextBox = ((RichTextLib::IRichTextPtr)(var_Editor->GetUserEditorObject()));
var_RichTextBox->PutAutoVerbMenu(VARIANT_TRUE);
var_RichTextBox->PutTextRTF(L"{\\rtf1\\ansi{\\fonttbl\\f0\\fswiss Helvetica;}\\f0\\pard\\r\\nThis is some {\\b bold} text.\\par\\r\\n}");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("RICHTEXT.RichtextCtrl");
spGrid1->EndUpdate();
|
992
|
Is it possible to trap a double-click event on a specific cell and when that happens, to set the cell to a specific value
// DblClick event - Occurs when the user dblclk the left mouse button over an object.
void OnDblClickGrid1(short Shift,long X,long Y)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
long h = spGrid1->GetItemFromPoint(-1,-1,c,hit);
OutputDebugStringW( _bstr_t(spGrid1->GetItems()->GetCellValue(h,c)) );
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutHeaderAppearance(EXGRIDLib::Etched);
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
spGrid1->GetColumns()->Add(L"C1");
spGrid1->GetColumns()->Add(L"C2");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(var_Items->AddItem("Item 1"),long(1),"Item 2");
var_Items->PutCellValue(var_Items->AddItem("Item 3"),long(1),"Item 4");
var_Items->PutCellValue(var_Items->AddItem("Item 5"),long(1),"Item 6");
spGrid1->EndUpdate();
|
991
|
How can I display dates in DD/MM/YYYY format
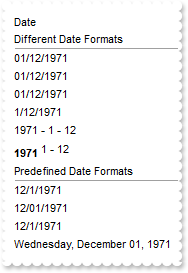
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutScrollBySingleLine(VARIANT_FALSE);
spGrid1->GetColumns()->Add(L"Date");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutItemDivider(var_Items->AddItem("Different Date Formats"),0);
var_Items->PutFormatCell(var_Items->AddItem(COleDateTime(1971,12,1,0,00,00).operator DATE()),long(0),L"((shortdateF(value) mid 4) left 2) + `/` + (shortdateF (value) left 2) + `/` + (shortdateF (value) right 4)");
var_Items->PutFormatCell(var_Items->AddItem(COleDateTime(1971,12,1,0,00,00).operator DATE()),long(0),L"(1 array (0:=(shortdateF(value) split `/`))) + `/` + (0 array (=:0) ) + `/` + (2 array (=:0) )");
var_Items->PutFormatCell(var_Items->AddItem(COleDateTime(1971,12,1,0,00,00).operator DATE()),long(0),L"((`0` + day(value) ) right 2) + `/` + ((`0` + month(value) ) right 2) + `/` + year(value)");
var_Items->PutFormatCell(var_Items->AddItem(COleDateTime(1971,12,1,0,00,00).operator DATE()),long(0),L"day(value) + `/` + month(value) + `/` + year(value)");
var_Items->PutFormatCell(var_Items->AddItem(COleDateTime(1971,12,1,0,00,00).operator DATE()),long(0),L"year(value) + ` - ` + day(value) + ` - ` + month(value)");
long h = var_Items->AddItem(COleDateTime(1971,12,1,0,00,00).operator DATE());
var_Items->PutItemHeight(h,24);
var_Items->PutCellValueFormat(h,long(0),EXGRIDLib::exHTML);
var_Items->PutFormatCell(h,long(0),L"`<b>` + year(value) + `</b><off -4> ` + day(value) + ` - ` + month(value)");
var_Items->PutItemDivider(var_Items->AddItem("Predefined Date Formats"),0);
var_Items->PutFormatCell(var_Items->AddItem(COleDateTime(1971,12,1,0,00,00).operator DATE()),long(0),L"value");
var_Items->PutFormatCell(var_Items->AddItem(COleDateTime(1971,12,1,0,00,00).operator DATE()),long(0),L"shortdateF(value)");
var_Items->PutFormatCell(var_Items->AddItem(COleDateTime(1971,12,1,0,00,00).operator DATE()),long(0),L"shortdate(value)");
var_Items->PutFormatCell(var_Items->AddItem(COleDateTime(1971,12,1,0,00,00).operator DATE()),long(0),L"longdate(value)");
spGrid1->EndUpdate();
|
990
|
I have noticed that the column gets resized once I release the mouse. I have a column that displays multiple-lines cells, and the text gets wrapped only when user releases the mouse. Is it possible to get resized contiguously as I had before
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutScrollBySingleLine(VARIANT_FALSE);
spGrid1->PutDrawGridLines(EXGRIDLib::exVLines);
spGrid1->PutColumnsAllowSizing(VARIANT_TRUE);
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Column A (cont)")))->PutDef(EXGRIDLib::exColumnResizeContiguously,VARIANT_TRUE);
spGrid1->GetColumns()->Add(L"Column 1");
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Column B (cont)")))->PutDef(EXGRIDLib::exColumnResizeContiguously,VARIANT_TRUE);
spGrid1->GetColumns()->Add(L"Column 2");
spGrid1->EndUpdate();
|
989
|
How do I get the column from cursor, when it hovers the empty portion of the items section
// MouseMove event - Occurs when the user moves the mouse.
void OnMouseMoveGrid1(short Button,short Shift,long X,long Y)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
long i = spGrid1->GetItemFromPoint(0,-1,c,hit);
OutputDebugStringW( L"Column" );
OutputDebugStringW( L"c" );
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutDrawGridLines(EXGRIDLib::exVLines);
spGrid1->GetColumns()->Add(L"Column 0");
spGrid1->GetColumns()->Add(L"Column 1");
spGrid1->GetColumns()->Add(L"Column 2");
spGrid1->EndUpdate();
|
988
|
How do I add items once the user clicks the empty area
// Click event - Occurs when the user presses and then releases the left mouse button over the grid control.
void OnClickGrid1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
long i = spGrid1->GetItemFromPoint(0,-1,c,hit);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(var_Items->AddItem(i),long(1),c);
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->GetColumns()->Add(L"Number of Items to Add");
spGrid1->GetColumns()->Add(L"Click on Column");
spGrid1->EndUpdate();
|
987
|
Is there any option to stop events
// AddItem event - Occurs after a new Item has been inserted to Items collection.
void OnAddItemGrid1(long Item)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
OutputDebugStringW( L"AddItem event is fired only if FreezeEvents(False) is called" );
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->FreezeEvents(VARIANT_TRUE);
spGrid1->BeginUpdate();
spGrid1->PutDefaultItemHeight(24);
spGrid1->GetColumns()->Add(L"Task");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h1 = var_Items->AddItem("Task 1");
long h2 = var_Items->AddItem("Task 2");
spGrid1->EndUpdate();
spGrid1->FreezeEvents(VARIANT_FALSE);
|
986
|
How can I include the child items, when a filter is applied
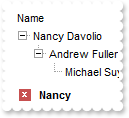
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_TRUE);
spGrid1->PutContinueColumnScroll(VARIANT_FALSE);
spGrid1->PutMarkSearchColumn(VARIANT_FALSE);
spGrid1->PutSearchColumnIndex(1);
spGrid1->PutIndent(16);
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
spGrid1->PutFilterBarPromptVisible(EXGRIDLib::exFilterBarPromptVisible);
spGrid1->PutFilterBarPromptPattern(L"Nancy");
spGrid1->PutFilterInclude(EXGRIDLib::exItemsWithChilds);
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellValue(h0,long(1),"Sales Representative");
var_Items->PutCellValue(h0,long(2),"Seattle");
h0 = var_Items->InsertItem(h0,vtMissing,"Andrew Fuller");
var_Items->PutCellValue(h0,long(1),"Vice President, Sales");
var_Items->PutCellValue(h0,long(2),"Tacoma");
h0 = var_Items->InsertItem(h0,vtMissing,"Michael Suyama");
var_Items->PutCellValue(h0,long(1),"Sales Representative");
var_Items->PutCellValue(h0,long(2),"London");
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellValue(h0,long(1),"Sales Representative");
var_Items->PutCellValue(h0,long(2),"Kirkland");
h0 = var_Items->InsertItem(h0,vtMissing,"Margaret Peacock");
var_Items->PutCellValue(h0,long(1),"Sales Representative");
var_Items->PutCellValue(h0,long(2),"Redmond");
var_Items->PutExpandItem(0,VARIANT_TRUE);
spGrid1->ApplyFilter();
spGrid1->EndUpdate();
|
985
|
How do I prevent changing the cell's state ( check-box state )
// CellStateChanging event - Fired before cell's state is about to be changed.
void OnCellStateChangingGrid1(long Item,long ColIndex,long FAR* NewState)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
NewState = var_Items->GetCellState(Item,ColIndex);
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"P1")));
var_Column->PutDef(EXGRIDLib::exCellHasCheckBox,VARIANT_TRUE);
var_Column->PutPartialCheck(VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"P2")));
var_Column1->PutDef(EXGRIDLib::exCellHasCheckBox,VARIANT_TRUE);
var_Column1->PutPartialCheck(VARIANT_TRUE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
spGrid1->EndUpdate();
|
984
|
How do I get sorted the column as string, numeric, date, date and time. Also how can it be applied to drop down filter panel
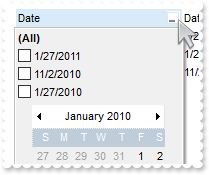
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Date")));
var_Column->PutSortType(EXGRIDLib::SortDate);
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column->PutDisplayFilterDate(VARIANT_TRUE);
var_Column->PutFilterList(EXGRIDLib::FilterListEnum(EXGRIDLib::exShowFocusItem | EXGRIDLib::exShowCheckBox | EXGRIDLib::exSortItemsDesc));
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"DateTime")));
var_Column1->PutSortType(EXGRIDLib::SortDateTime);
var_Column1->PutDisplayFilterButton(VARIANT_TRUE);
var_Column1->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column1->PutFilterList(EXGRIDLib::FilterListEnum(EXGRIDLib::exShowFocusItem | EXGRIDLib::exShowCheckBox | EXGRIDLib::exSortItemsDesc));
EXGRIDLib::IColumnPtr var_Column2 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Time")));
var_Column2->PutSortType(EXGRIDLib::SortTime);
var_Column2->PutDisplayFilterButton(VARIANT_TRUE);
var_Column2->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column2->PutFilterList(EXGRIDLib::FilterListEnum(EXGRIDLib::exShowFocusItem | EXGRIDLib::exShowCheckBox | EXGRIDLib::exSortItemsDesc));
var_Column2->PutFormatColumn(L"time(value)");
EXGRIDLib::IColumnPtr var_Column3 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Numeric")));
var_Column3->PutSortType(EXGRIDLib::SortNumeric);
var_Column3->PutDisplayFilterButton(VARIANT_TRUE);
var_Column3->PutFilterList(EXGRIDLib::FilterListEnum(EXGRIDLib::exShowFocusItem | EXGRIDLib::exShowCheckBox | EXGRIDLib::exSortItemsDesc));
EXGRIDLib::IColumnPtr var_Column4 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"String")));
var_Column4->PutDisplayFilterButton(VARIANT_TRUE);
var_Column4->PutFilterList(EXGRIDLib::FilterListEnum(EXGRIDLib::exShowFocusItem | EXGRIDLib::exShowCheckBox | EXGRIDLib::exSortItemsDesc));
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem(COleDateTime(2010,1,27,0,00,00).operator DATE());
var_Items->PutCellValue(h,long(1),COleDateTime(2010,1,27,10,00,00).operator DATE());
var_Items->PutCellValue(h,long(2),var_Items->GetCellValue(h,long(1)));
var_Items->PutCellValue(h,long(3),long(1));
var_Items->PutCellValue(h,long(4),var_Items->GetCellValue(h,long(3)));
h = var_Items->AddItem(COleDateTime(2011,1,27,0,00,00).operator DATE());
var_Items->PutCellValue(h,long(1),COleDateTime(2011,1,27,9,00,00).operator DATE());
var_Items->PutCellValue(h,long(2),var_Items->GetCellValue(h,long(1)));
var_Items->PutCellValue(h,long(3),long(11));
var_Items->PutCellValue(h,long(4),var_Items->GetCellValue(h,long(3)));
h = var_Items->AddItem(COleDateTime(2010,11,2,0,00,00).operator DATE());
var_Items->PutCellValue(h,long(1),COleDateTime(2010,11,2,9,00,00).operator DATE());
var_Items->PutCellValue(h,long(2),var_Items->GetCellValue(h,long(1)));
var_Items->PutCellValue(h,long(3),long(2));
var_Items->PutCellValue(h,long(4),var_Items->GetCellValue(h,long(3)));
spGrid1->GetColumns()->GetItem("DateTime")->PutDisplayFilterDate(VARIANT_FALSE);
spGrid1->EndUpdate();
|
983
|
I am using Layout property to sort multiple columns at once. The problem is that all items get expanded. How do I prevent that
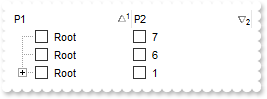
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"P1")));
var_Column->PutDef(EXGRIDLib::exCellHasCheckBox,VARIANT_TRUE);
var_Column->PutPartialCheck(VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"P2")));
var_Column1->PutDef(EXGRIDLib::exCellHasCheckBox,VARIANT_TRUE);
var_Column1->PutPartialCheck(VARIANT_TRUE);
var_Column1->PutFormatColumn(L"1 index ``");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child A");
var_Items->InsertItem(h,vtMissing,"Child B");
var_Items->InsertItem(h,vtMissing,"Child A");
var_Items->InsertItem(h,vtMissing,"Child B");
var_Items->AddItem("Root");
var_Items->AddItem("Root");
spGrid1->PutSingleSort(VARIANT_FALSE);
spGrid1->PutLayout(L"multiplesort=\"C0:1 C1:2\";collapse=\"\"");
spGrid1->EndUpdate();
|
982
|
How do I find the cell's type, or what the cell holds
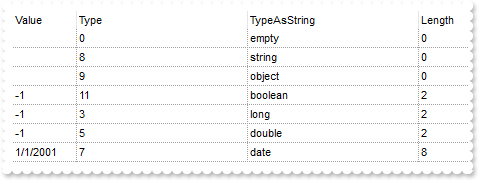
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Value")))->PutWidth(24);
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Type")))->PutFormatColumn(L"type(%0)");
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"TypeAsString")))->PutFormatColumn(_bstr_t("(0 := type(%0)) array (`empty`, `null`, `short`, `long`, `float`, `double`, `currency`, `date`, `string`, `object`, `error`, `b") +
"oolean`, `variant`, `any`, `reserved`, `decimal`, `char`, `byte`, `unsigned short`, `unsigned long`, `long on 64 bits`)");
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Length")))->PutFormatColumn(L"len(%0)");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem(vtMissing);
var_Items->AddItem("");
var_Items->PutCellValue(->AddItem(vtMissing),long(0),((EXGRIDLib::IGridPtr)(spGrid1)));
var_Items->PutCellValue(var_Items->AddItem(vtMissing),long(0),VARIANT_TRUE);
var_Items->PutCellValue(var_Items->AddItem(vtMissing),long(0),long(-1));
var_Items->PutCellValue(var_Items->AddItem(vtMissing),long(0),long(-1));
var_Items->PutCellValue(var_Items->AddItem(vtMissing),long(0),COleDateTime(2001,1,1,0,00,00).operator DATE());
spGrid1->EndUpdate();
|
981
|
How can I get ride / hide the image being dragged by OLE Drag and Drop
// OLEStartDrag event - Occurs when the OLEDrag method is called.
void OnOLEStartDragGrid1(LPDISPATCH Data,long FAR* AllowedEffects)
{
// Data.SetData("data to drag")
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
AllowedEffects = 1;
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->PutOLEDropMode(EXGRIDLib::exOLEDropManual);
spGrid1->PutBackground(EXGRIDLib::exDragDropAfter,RGB(255,255,255));
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
spGrid1->GetColumns()->Add(L"Default");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
980
|
The ReadOnly property does not prevent changing the column's check-box (sample 2)
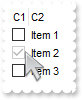
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutShowFocusRect(VARIANT_FALSE);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"C1")));
var_Column->PutAllowSizing(VARIANT_FALSE);
var_Column->PutWidth(18);
var_Column->GetEditor()->PutEditType(EXGRIDLib::CheckValueType);
spGrid1->GetColumns()->Add(L"C2");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(var_Items->AddItem(long(0)),long(1),"Item 1");
var_Items->PutCellValue(var_Items->AddItem(long(-1)),long(1),"Item 2");
var_Items->PutCellValue(var_Items->AddItem(long(0)),long(1),"Item 3");
spGrid1->PutReadOnly(EXGRIDLib::exReadOnly);
spGrid1->GetColumns()->GetItem(long(0))->GetEditor()->PutOption(EXGRIDLib::exCheckValue2,long(2));
spGrid1->EndUpdate();
|
979
|
The ReadOnly property does not prevent changing the column's check-box (sample 1)
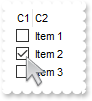
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutShowFocusRect(VARIANT_FALSE);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"C1")));
var_Column->PutAllowSizing(VARIANT_FALSE);
var_Column->PutWidth(18);
EXGRIDLib::IEditorPtr var_Editor = var_Column->GetEditor();
var_Editor->PutEditType(EXGRIDLib::CheckValueType);
var_Editor->PutOption(EXGRIDLib::exCheckValue2,long(1));
spGrid1->GetColumns()->Add(L"C2");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(var_Items->AddItem(long(0)),long(1),"Item 1");
var_Items->PutCellValue(var_Items->AddItem(long(-1)),long(1),"Item 2");
var_Items->PutCellValue(var_Items->AddItem(long(0)),long(1),"Item 3");
spGrid1->PutReadOnly(EXGRIDLib::exReadOnly);
spGrid1->EndUpdate();
|
978
|
How can I export checked items only
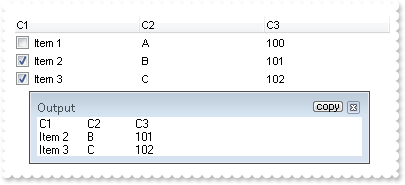
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C1")))->PutDef(EXGRIDLib::exCellHasCheckBox,VARIANT_TRUE);
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C2")))->PutFormatColumn(L"1 index `A-Z`");
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C3")))->PutFormatColumn(L"100 index ``");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("Item 1");
var_Items->PutCellState(var_Items->AddItem("Item 2"),vtMissing,1);
var_Items->PutCellState(var_Items->AddItem("Item 3"),vtMissing,1);
spGrid1->EndUpdate();
OutputDebugStringW( L"Export CSV Checked Items Only:" );
OutputDebugStringW( _bstr_t(spGrid1->Export("","chk")) );
|
977
|
How can I export a hidden column
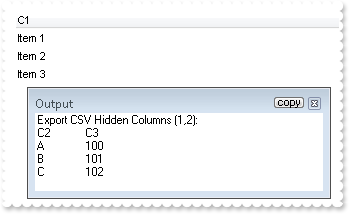
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
var_Columns->Add(L"C1");
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C2")));
var_Column->PutFormatColumn(L"1 index `A-Z`");
var_Column->PutVisible(VARIANT_FALSE);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C3")));
var_Column1->PutFormatColumn(L"100 index ``");
var_Column1->PutVisible(VARIANT_FALSE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
var_Items->AddItem("Item 3");
spGrid1->EndUpdate();
OutputDebugStringW( L"Export CSV Hidden Columns (1,2):" );
OutputDebugStringW( _bstr_t(spGrid1->Export("","|1,2")) );
|
976
|
I'm trying to use automatic numbering of the outline. How can I have A, B, C for root items, 1, 2, 3 for the sub-items, and a, b, c for sub-sub-items (sample 3)
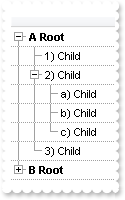
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
spGrid1->PutAutoDrag(EXGRIDLib::exAutoDragPositionAny);
spGrid1->PutHasLines(EXGRIDLib::exSolidLine);
spGrid1->PutIndent(16);
spGrid1->PutMarkSearchColumn(VARIANT_FALSE);
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"")));
var_Column->PutDef(EXGRIDLib::exCellValueFormat,long(1));
var_Column->PutFormatColumn(L"((1:=(0 :=(1 rpos '.|A-Z||a-z|')) rfind `.`) < 0 ? `<b>` + =:0 + `` : (=:0 mid (1 + 1 + =:1) ) + `)` ) + ` ` + value");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child");
long hChild = var_Items->InsertItem(h,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(h,vtMissing,"Child");
var_Items->PutExpandItem(0,VARIANT_TRUE);
h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child");
hChild = var_Items->InsertItem(h,vtMissing,"Child");
var_Items->PutCellState(hChild,long(0),1);
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(h,vtMissing,"Child");
spGrid1->EndUpdate();
|
975
|
I'm trying to use automatic numbering of the outline. How can I have A, B, C for root items, 1, 2, 3 for the sub-items, and a, b, c for sub-sub-items (sample 2)
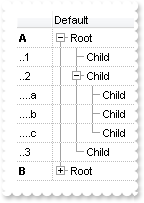
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
spGrid1->PutGridLineColor(RGB(190,190,190));
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
spGrid1->PutAutoDrag(EXGRIDLib::exAutoDragPositionAny);
spGrid1->PutHasLines(EXGRIDLib::exSolidLine);
spGrid1->PutIndent(16);
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
var_Columns->Add(L"Default");
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"")));
var_Column->PutDef(EXGRIDLib::exCellValueFormat,long(1));
var_Column->PutDef(EXGRIDLib::exCellPaddingRight,long(4));
var_Column->PutAllowSizing(VARIANT_FALSE);
var_Column->PutWidth(36);
var_Column->PutPosition(0);
var_Column->PutFormatColumn(_bstr_t("(1:=(0 :=(1 rpos '.|A-Z||a-z|')) rfind `.`) < 0 ? `<b>` + =:0 : (`............` left 2 * (=:0 count `.`)) + (=:0 mid (1 + 1 + =") +
":1) ) ");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child");
long hChild = var_Items->InsertItem(h,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(h,vtMissing,"Child");
var_Items->PutExpandItem(0,VARIANT_TRUE);
h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child");
hChild = var_Items->InsertItem(h,vtMissing,"Child");
var_Items->PutCellState(hChild,long(0),1);
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(h,vtMissing,"Child");
spGrid1->EndUpdate();
|
974
|
I'm trying to use automatic numbering of the outline. How can I have A, B, C for root items, 1, 2, 3 for the sub-items, and a, b, c for sub-sub-items (sample 1)
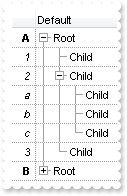
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
spGrid1->PutAutoDrag(EXGRIDLib::exAutoDragPositionAny);
spGrid1->PutHasLines(EXGRIDLib::exSolidLine);
spGrid1->PutIndent(16);
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
var_Columns->Add(L"Default");
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"")));
var_Column->PutDef(EXGRIDLib::exCellValueFormat,long(1));
var_Column->PutDef(EXGRIDLib::exCellPaddingRight,long(4));
var_Column->PutAlignment(EXGRIDLib::RightAlignment);
var_Column->PutAllowSizing(VARIANT_FALSE);
var_Column->PutWidth(24);
var_Column->PutPosition(0);
var_Column->PutFormatColumn(L"(1:=(0 :=(1 rpos '.|A-Z||a-z|')) rfind `.`) < 0 ? `<b>` + =:0 : `<i>` + (=:0 mid (1 + 1 + =:1) ) ");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child");
long hChild = var_Items->InsertItem(h,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(h,vtMissing,"Child");
var_Items->PutExpandItem(0,VARIANT_TRUE);
h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child");
hChild = var_Items->InsertItem(h,vtMissing,"Child");
var_Items->PutCellState(hChild,long(0),1);
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(hChild,vtMissing,"Child");
var_Items->InsertItem(h,vtMissing,"Child");
spGrid1->EndUpdate();
|
973
|
How can I programmatically group by columns, without having the control's sort bar visible
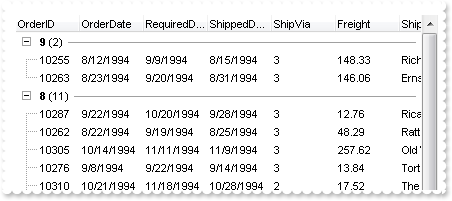
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutSortBarHeight(0);
spGrid1->PutSortBarVisible(VARIANT_TRUE);
spGrid1->PutSortBarCaption(L"Drag a <b>column</b> header here to group by that column.");
spGrid1->PutAllowGroupBy(VARIANT_TRUE);
spGrid1->PutLayout(L"multiplesort=\"C1:2\"");
spGrid1->EndUpdate();
|
972
|
How do I perform my own sort
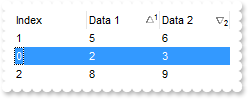
// Sort event - Fired when the control sorts a column.
void OnSortGrid1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
OutputDebugStringW( L"Sort" );
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutItemPosition(var_Items->GetItemByIndex(1),0);
var_Items->PutItemPosition(var_Items->GetItemByIndex(0),1);
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutSingleSort(VARIANT_FALSE);
spGrid1->PutSortOnClick(EXGRIDLib::exUserSort);
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Index")))->PutFormatColumn(L"0 index ``");
var_Columns->Add(L"Data 1");
var_Columns->Add(L"Data 2");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem(long(0));
var_Items->PutCellValue(h,long(1),long(2));
var_Items->PutCellValue(h,long(2),long(3));
h = var_Items->AddItem(long(4));
var_Items->PutCellValue(h,long(1),long(5));
var_Items->PutCellValue(h,long(2),long(6));
h = var_Items->AddItem(long(7));
var_Items->PutCellValue(h,long(1),long(8));
var_Items->PutCellValue(h,long(2),long(9));
spGrid1->PutLayout(L"multiplesort=\"C1:1 C2:2\"");
spGrid1->EndUpdate();
|
971
|
Is it possible to have a different alignment for parts of the cell's caption
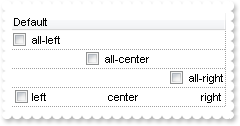
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutTreeColumnIndex(-1);
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Default")));
var_Column->PutDef(EXGRIDLib::exCellHasCheckBox,VARIANT_TRUE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellHAlignment(var_Items->AddItem("all-left"),long(0),EXGRIDLib::LeftAlignment);
var_Items->PutCellHAlignment(var_Items->AddItem("all-center"),long(0),EXGRIDLib::CenterAlignment);
var_Items->PutCellHAlignment(var_Items->AddItem("all-right"),long(0),EXGRIDLib::RightAlignment);
long h = var_Items->AddItem("left<c>center<r>right");
var_Items->PutCellValueFormat(h,long(0),EXGRIDLib::exHTML);
spGrid1->EndUpdate();
|
970
|
I have a column with Def(exCellSingleLine) property on False, word-wrapping, and I am wondering if possible to update the column's content while user is resizing it
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"MultipleLine")));
var_Column->PutWidth(32);
var_Column->PutDef(EXGRIDLib::exCellSingleLine,VARIANT_FALSE);
var_Column->PutDef(EXGRIDLib::exColumnResizeContiguously,VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"SingleLine")));
var_Column1->PutDef(EXGRIDLib::exCellSingleLine,VARIANT_FALSE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(var_Items->AddItem("This is a bit of long text that should break the line"),long(1),"This is a bit of long text that should break the line");
spGrid1->EndUpdate();
|
969
|
How can I get the absolute position of an item
// MouseMove event - Occurs when the user moves the mouse.
void OnMouseMoveGrid1(short Button,short Shift,long X,long Y)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
OutputDebugStringW( var_Items->GetCellCaption(spGrid1->GetItemFromPoint(-1,-1,c,hit),"Position") );
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutBackColorAlternate(RGB(240,240,240));
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Def")))->PutDisplayFilterButton(VARIANT_TRUE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(var_Items->InsertItem(h,vtMissing,"Child 1"),vtMissing,"Sub-Child 1");
var_Items->InsertItem(var_Items->InsertItem(h,vtMissing,"Child 2"),vtMissing,"Sub-Child 2");
spGrid1->PutItems(spGrid1->GetItems(long(-1)),vtMissing);
spGrid1->PutItems(spGrid1->GetItems(long(-1)),vtMissing);
spGrid1->PutItems(spGrid1->GetItems(long(-1)),vtMissing);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Position")));
var_Column->PutFormatColumn(L"1 apos ``");
var_Column->PutVisible(VARIANT_FALSE);
spGrid1->EndUpdate();
|
968
|
I am using ExComboBox as an user editor, how can I display a different column
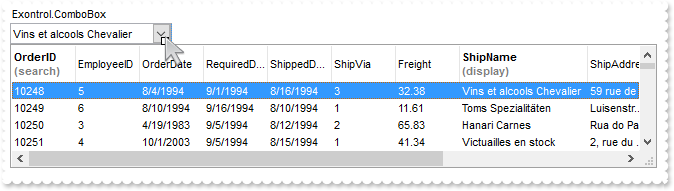
// UserEditorClose event - Fired the user editor is about to be opened.
void OnUserEditorCloseGrid1(LPDISPATCH Object,long Item,long ColIndex)
{
// Items.CellValue(Item,ColIndex) = Object.Value
}
// UserEditorOleEvent event - Occurs when an user editor fires an event.
void OnUserEditorOleEventGrid1(LPDISPATCH Object,LPDISPATCH Ev,BOOL FAR* CloseEditor,long Item,long ColIndex)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
OutputDebugStringW( L"Ev" );
}
// UserEditorOpen event - Occurs when an user editor is about to be opened.
void OnUserEditorOpenGrid1(LPDISPATCH Object,long Item,long ColIndex)
{
// Object.Value = Me.Items.CellValue(Item,ColIndex)
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IEditorPtr var_Editor = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Exontrol.ComboBox")))->GetEditor();
var_Editor->PutEditType(EXGRIDLib::UserEditorType);
var_Editor->UserEditor(L"Exontrol.ComboBox",L"");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXCOMBOBOXLib' for the library: 'ExComboBox 1.0 Control Library'
#import <ExComboBox.dll>
using namespace EXCOMBOBOXLib;
*/
EXCOMBOBOXLib::IComboBoxPtr var_ComboBox = ((EXCOMBOBOXLib::IComboBoxPtr)(var_Editor->GetUserEditorObject()));
var_ComboBox->BeginUpdate();
var_ComboBox->PutStyle(EXCOMBOBOXLib::DropDownList);
var_ComboBox->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
var_ComboBox->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
var_ComboBox->PutAlignment(EXCOMBOBOXLib::LeftAlignment);
var_ComboBox->PutIntegralHeight(VARIANT_TRUE);
var_ComboBox->PutMinHeightList(128);
var_ComboBox->PutMinWidthList(648);
var_ComboBox->PutHeaderHeight(36);
var_ComboBox->PutAllowSizeGrip(VARIANT_TRUE);
var_ComboBox->PutSingleEdit(VARIANT_TRUE);
var_ComboBox->PutLabelColumnIndex(7);
var_ComboBox->PutSearchColumnIndex(0);
EXCOMBOBOXLib::IColumnPtr var_Column = var_ComboBox->GetColumns()->GetItem(long(0));
var_Column->PutHeaderBold(VARIANT_TRUE);
var_Column->PutHTMLCaption(L"OrderID<br><fgcolor=808080>(search)");
EXCOMBOBOXLib::IColumnPtr var_Column1 = var_ComboBox->GetColumns()->GetItem(long(7));
var_Column1->PutHeaderBold(VARIANT_TRUE);
var_Column1->PutHTMLCaption(L"ShipName<br><fgcolor=808080>(display)");
var_Column1->PutWidth(128);
var_ComboBox->PutUseTabKey(VARIANT_FALSE);
var_ComboBox->EndUpdate();
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
spGrid1->PutDefaultItemHeight(21);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellEditorVisible(var_Items->AddItem(long(10248)),long(0),EXGRIDLib::exEditorVisible);
var_Items->PutCellEditorVisible(var_Items->AddItem(long(10249)),long(0),EXGRIDLib::exEditorVisible);
var_Items->PutCellEditorVisible(var_Items->AddItem(long(10250)),long(0),EXGRIDLib::exEditorVisible);
spGrid1->EndUpdate();
|
967
|
How do I sort the index column as numeric (Method 3)
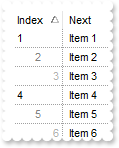
// AddItem event - Occurs after a new Item has been inserted to Items collection.
void OnAddItemGrid1(long Item)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellData(Item,long(1),var_Items->GetCellCaption(Item,long(1)));
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutDrawGridLines(EXGRIDLib::exAllLines);
spGrid1->PutColumnAutoResize(VARIANT_TRUE);
spGrid1->PutShowFocusRect(VARIANT_FALSE);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Next")));
var_Column->PutDef(EXGRIDLib::exCellPaddingLeft,long(4));
var_Column->PutDef(EXGRIDLib::exHeaderPaddingLeft,long(4));
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Index")));
var_Column1->PutAllowSizing(VARIANT_FALSE);
var_Column1->PutWidth(48);
var_Column1->PutFormatColumn(L"(((0 := (1 index ``)) mod 3) case ( default: ``; 0 : `<r><fgcolor=B0B0B0>`; 1: ``; 2 : `<c><fgcolor=808080>` )) + str(=:0)");
var_Column1->PutDef(EXGRIDLib::exCellValueFormat,long(1));
var_Column1->PutSortType(EXGRIDLib::SortUserData);
var_Column1->PutPosition(0);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 4");
var_Items->AddItem("Item 5");
var_Items->AddItem("Item 6");
var_Items->AddItem("Item 7");
var_Items->AddItem("Item 8");
var_Items->AddItem("Item 9");
var_Items->AddItem("Item 10");
spGrid1->EndUpdate();
|
966
|
How do I sort the index column as numeric (Method 2)
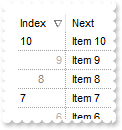
// AddItem event - Occurs after a new Item has been inserted to Items collection.
void OnAddItemGrid1(long Item)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellSortData(Item,long(1),var_Items->GetCellCaption(Item,long(1)));
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutDrawGridLines(EXGRIDLib::exAllLines);
spGrid1->PutColumnAutoResize(VARIANT_TRUE);
spGrid1->PutShowFocusRect(VARIANT_FALSE);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Next")));
var_Column->PutDef(EXGRIDLib::exCellPaddingLeft,long(4));
var_Column->PutDef(EXGRIDLib::exHeaderPaddingLeft,long(4));
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Index")));
var_Column1->PutAllowSizing(VARIANT_FALSE);
var_Column1->PutWidth(48);
var_Column1->PutFormatColumn(L"(((0 := (1 index ``)) mod 3) case ( default: ``; 0 : `<r><fgcolor=B0B0B0>`; 1: ``; 2 : `<c><fgcolor=808080>` )) + str(=:0)");
var_Column1->PutDef(EXGRIDLib::exCellValueFormat,long(1));
var_Column1->PutSortType(EXGRIDLib::SortCellData);
var_Column1->PutPosition(0);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 4");
var_Items->AddItem("Item 5");
var_Items->AddItem("Item 6");
var_Items->AddItem("Item 7");
var_Items->AddItem("Item 8");
var_Items->AddItem("Item 9");
var_Items->AddItem("Item 10");
spGrid1->EndUpdate();
|
965
|
How do I sort the index column as numeric (Method 1)
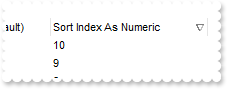
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Sort Index As String (Default)")));
var_Column->PutFormatColumn(L"1 index ``");
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Sort Index As Numeric")));
var_Column1->PutComputedField(L"%C0");
var_Column1->PutSortType(EXGRIDLib::SortNumeric);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
spGrid1->EndUpdate();
|
964
|
How can I put icons/images into buttons
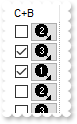
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_TRUE);
spGrid1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"C+B")));
var_Column->PutAllowSizing(VARIANT_FALSE);
var_Column->PutWidth(48);
var_Column->PutFormatColumn(L"` <img>` + ( 1 + (1 index ``) mod 3 ) + `</img> `");
var_Column->PutDef(EXGRIDLib::exCellValueFormat,long(1));
var_Column->PutDef(EXGRIDLib::exCellHasCheckBox,VARIANT_TRUE);
var_Column->PutDef(EXGRIDLib::exCellHasButton,VARIANT_TRUE);
var_Column->PutDef(EXGRIDLib::exCellButtonAutoWidth,VARIANT_TRUE);
spGrid1->GetColumns()->Add(L"");
spGrid1->PutDrawGridLines(EXGRIDLib::exVLines);
spGrid1->PutDefaultItemHeight(20);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
spGrid1->EndUpdate();
|
963
|
Is it possible to have a CheckBox and Button TOGETHER on all cells in a column
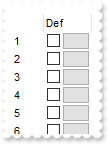
// ButtonClick event - Occurs when user clicks on the cell's button.
void OnButtonClickGrid1(long Item,long ColIndex,VARIANT Key)
{
OutputDebugStringW( L"ButtonClick" );
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
OutputDebugStringW( L"Item" );
OutputDebugStringW( L"Key" );
}
// CellStateChanged event - Fired after cell's state has been changed.
void OnCellStateChangedGrid1(long Item,long ColIndex)
{
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
OutputDebugStringW( L"CellStateChanged" );
OutputDebugStringW( L"Item" );
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"")));
var_Column->PutAllowSizing(VARIANT_FALSE);
var_Column->PutWidth(32);
var_Column->PutFormatColumn(L"1 index ``");
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Def")));
var_Column1->PutAllowSizing(VARIANT_FALSE);
var_Column1->PutWidth(48);
var_Column1->PutFormatColumn(L"` `");
var_Column1->PutDef(EXGRIDLib::exCellHasCheckBox,VARIANT_TRUE);
var_Column1->PutDef(EXGRIDLib::exCellHasButton,VARIANT_TRUE);
var_Column1->PutDef(EXGRIDLib::exCellButtonAutoWidth,VARIANT_TRUE);
spGrid1->GetColumns()->Add(L"");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
spGrid1->EndUpdate();
|
962
|
I have columns that look up the same data. (e.g. different contact) so both could / should use the same editor. Is this possible, to use other column's editor
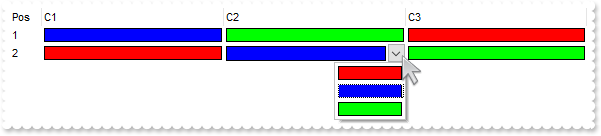
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutSelBackColor(spGrid1->GetBackColor());
spGrid1->PutSelForeColor(spGrid1->GetForeColor());
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Pos")));
var_Column->PutWidth(32);
var_Column->PutAllowSizing(VARIANT_FALSE);
var_Column->PutFormatColumn(L"1 index ``");
EXGRIDLib::IEditorPtr var_Editor = var_Columns->Add(L"C1")->GetEditor();
var_Editor->PutEditType(EXGRIDLib::ColorListType);
var_Editor->ClearItems();
var_Editor->AddItem(255,L"Red Color",vtMissing);
var_Editor->AddItem(16711680,L"Blue Color",vtMissing);
var_Editor->AddItem(65280,L"Green Color",vtMissing);
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C2")))->GetEditor()->PutEditType(EXGRIDLib::EditTypeEnum(EXGRIDLib::CloneType | EXGRIDLib::EditType));
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C3")))->GetEditor()->PutEditType(EXGRIDLib::EditTypeEnum(EXGRIDLib::CloneType | EXGRIDLib::EditType));
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("");
var_Items->PutCellValue(h,long(1),long(16711680));
var_Items->PutCellValue(h,long(2),long(65280));
var_Items->PutCellValue(h,long(3),long(255));
h = var_Items->AddItem("");
var_Items->PutCellValue(h,long(1),long(255));
var_Items->PutCellValue(h,long(2),long(16711680));
var_Items->PutCellValue(h,long(3),long(65280));
spGrid1->EndUpdate();
|
961
|
Is there an easy way to get an effect like in a Microsoft Access / SQL-Server Table view, where you can scroll-up till the last row containing data is displayed as top-row
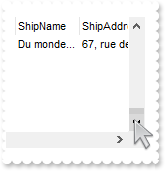
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutHasLines(EXGRIDLib::exNoLine);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutScrollBars(EXGRIDLib::ScrollBarsEnum(EXGRIDLib::exVScrollEmptySpace | EXGRIDLib::exBoth));
spGrid1->PutScrollPos(VARIANT_TRUE,spGrid1->GetItems()->GetItemCount());
spGrid1->EndUpdate();
|
960
|
Does filtering work with umlauts / accents characters
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Names")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutFilterType(EXGRIDLib::exPattern);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("Mantel");
var_Items->AddItem("Mechanik");
var_Items->AddItem("Motor");
var_Items->AddItem("Murks");
var_Items->AddItem("Märchen");
var_Items->AddItem("Möhren");
var_Items->AddItem("Mühle");
var_Items->AddItem("Sérigraphie");
spGrid1->GetColumns()->GetItem(long(0))->PutFilter(L"*ä*");
spGrid1->ApplyFilter();
spGrid1->EndUpdate();
|
959
|
How FullPath method works
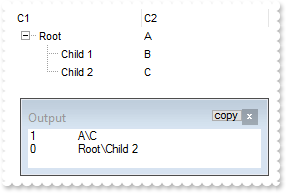
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
spGrid1->GetColumns()->Add(L"C1");
spGrid1->GetColumns()->Add(L"C2");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->PutCellValue(h,long(1),"A");
var_Items->PutCellValue(var_Items->InsertItem(h,vtMissing,"Child 1"),long(1),"B");
var_Items->PutCellValue(var_Items->InsertItem(h,vtMissing,"Child 2"),long(1),"C");
var_Items->PutExpandItem(h,VARIANT_TRUE);
spGrid1->PutSearchColumnIndex(1);
OutputDebugStringW( _bstr_t(spGrid1->GetSearchColumnIndex()) );
OutputDebugStringW( ->GetFullPath(spGrid1->GetItems()->GetItemByIndex(2)) );
spGrid1->PutSearchColumnIndex(0);
OutputDebugStringW( _bstr_t(spGrid1->GetSearchColumnIndex()) );
OutputDebugStringW( ->GetFullPath(spGrid1->GetItems()->GetItemByIndex(2)) );
spGrid1->EndUpdate();
|
958
|
Can I set the search box / filterbarprompt to invisible, so I can use my own input and *string* via VBA
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_TRUE);
spGrid1->PutContinueColumnScroll(VARIANT_FALSE);
spGrid1->PutMarkSearchColumn(VARIANT_FALSE);
spGrid1->PutSearchColumnIndex(1);
spGrid1->PutFilterBarHeight(0);
spGrid1->PutFilterBarPromptVisible(EXGRIDLib::exFilterBarPromptVisible);
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Name")))->PutWidth(96);
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Title")))->PutWidth(96);
var_Columns->Add(L"City");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h0 = var_Items->AddItem("Nancy Davolio");
var_Items->PutCellValue(h0,long(1),"Sales Representative");
var_Items->PutCellValue(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Andrew Fuller");
var_Items->PutCellValue(h0,long(1),"Vice President, Sales");
var_Items->PutCellValue(h0,long(2),"Tacoma");
var_Items->PutSelectItem(h0,VARIANT_TRUE);
h0 = var_Items->AddItem("Janet Leverling");
var_Items->PutCellValue(h0,long(1),"Sales Representative");
var_Items->PutCellValue(h0,long(2),"Kirkland");
h0 = var_Items->AddItem("Margaret Peacock");
var_Items->PutCellValue(h0,long(1),"Sales Representative");
var_Items->PutCellValue(h0,long(2),"Redmond");
h0 = var_Items->AddItem("Steven Buchanan");
var_Items->PutCellValue(h0,long(1),"Sales Manager");
var_Items->PutCellValue(h0,long(2),"London");
h0 = var_Items->AddItem("Michael Suyama");
var_Items->PutCellValue(h0,long(1),"Sales Representative");
var_Items->PutCellValue(h0,long(2),"London");
h0 = var_Items->AddItem("Robert King");
var_Items->PutCellValue(h0,long(1),"Sales Representative");
var_Items->PutCellValue(h0,long(2),"London");
h0 = var_Items->AddItem("Laura Callahan");
var_Items->PutCellValue(h0,long(1),"Inside Sales Coordinator");
var_Items->PutCellValue(h0,long(2),"Seattle");
h0 = var_Items->AddItem("Anne Dodsworth");
var_Items->PutCellValue(h0,long(1),"Sales Representative");
var_Items->PutCellValue(h0,long(2),"London");
spGrid1->PutFilterBarPromptPattern(L"London");
spGrid1->EndUpdate();
|
957
|
How to load a hierarchy using the control's DataSource property (Parent-ID-Relation)
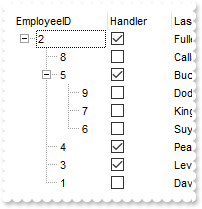
// AddItem event - Occurs after a new Item has been inserted to Items collection.
void OnAddItemGrid1(long Item)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->SetParent(Item,var_Items->GetFindItem(var_Items->GetCellValue(Item,"ReportsTo"),"EmployeeID",vtMissing));
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
spGrid1->PutContinueColumnScroll(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("SELECT * FROM Employees ORDER BY ReportsTo","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->GetItems()->PutExpandItem(0,VARIANT_TRUE);
spGrid1->EndUpdate();
|
956
|
Is it possible to select the entire row/line, when user clicks the first column, and select individually the rest of cells, while user clicks any other column
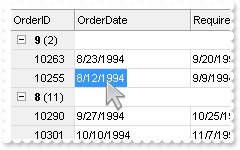
// MouseDown event - Occurs when the user presses a mouse button.
void OnMouseDownGrid1(short Button,short Shift,long X,long Y)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
long i = spGrid1->GetItemFromPoint(-1,-1,c,hit);
spGrid1->PutFullRowSelect(spGrid1->GetColumns()->GetItem(c)->GetData());
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutHeaderHeight(22);
spGrid1->PutHeaderAppearance(EXGRIDLib::Flat);
spGrid1->PutBackColorLock(RGB(240,240,240));
spGrid1->PutBackColorHeader(spGrid1->GetBackColorLock());
spGrid1->PutHasLines(EXGRIDLib::exNoLine);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
spGrid1->PutSortBarVisible(VARIANT_FALSE);
spGrid1->PutAllowGroupBy(VARIANT_TRUE);
spGrid1->PutReadOnly(EXGRIDLib::exReadOnly);
spGrid1->PutShowFocusRect(VARIANT_FALSE);
spGrid1->PutCountLockedColumns(1);
spGrid1->PutAutoDrag(EXGRIDLib::exAutoDragScroll);
spGrid1->PutSingleSort(VARIANT_FALSE);
spGrid1->PutColumnsAllowSizing(VARIANT_TRUE);
spGrid1->PutDrawGridLines(EXGRIDLib::exAllLines);
spGrid1->PutGridLineStyle(EXGRIDLib::exGridLinesSolid);
spGrid1->PutGridLineColor(RGB(220,220,220));
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->GetColumns()->GetItem(long(0))->PutData(long(-1));
spGrid1->PutLayout(L"singlesort=\"C5:1\";multiplesort=\" C1:2\"");
spGrid1->EndUpdate();
|
955
|
The user are not able to size the columns at runtime when using HeaderAppearance property on zero
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->GetVisualAppearance()->Add(1,_bstr_t("gBFLBCJwBAEHhEJAAEhABJEIQAAYAQGKIYBkAKBQAGaAoDDcMQ5QwAAyDGKEEwsACEIrjKCRShyCYZRhGcTSBCIZBqEqSZLiEZRQiiCYsS5GQBSFDcOwHGyQZonKK3L") +
"hGCYBgIA=");
spGrid1->PutHeaderAppearance(EXGRIDLib::AppearanceEnum(0x1000000));
spGrid1->GetColumns()->Add(L"1");
spGrid1->GetColumns()->Add(L"2");
spGrid1->GetColumns()->Add(L"3");
|
954
|
Is it possible to embed the exGauge into the exGrid control
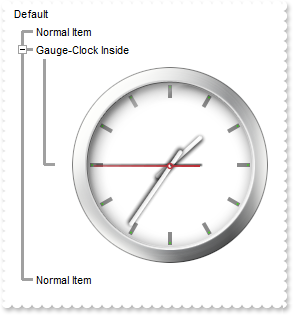
// ItemOleEvent event - Fired when an ActiveX control hosted by an item has fired an event.
void OnItemOleEventGrid1(long Item,LPDISPATCH Ev)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGAUGELib' for the library: 'ExGauge 1.0 Control Library'
#import <ExGauge.dll>
using namespace EXGAUGELib;
*/
EXGAUGELib::IGaugePtr var_Gauge = ((EXGAUGELib::IGaugePtr)(spGrid1->GetItems()->GetItemObject(spGrid1->GetItems()->GetItemByIndex(2))));
_variant_t v = var_Gauge->FormatABC(L"date(`now`)",vtMissing,vtMissing,vtMissing);
var_Gauge->GetLayers()->GetItem("sec")->PutValue(v);
var_Gauge->GetLayers()->GetItem("min")->PutValue(v);
var_Gauge->GetLayers()->GetItem("hour")->PutValue(v);
}
// MouseMove event - Occurs when the user moves the mouse.
void OnMouseMoveGrid1(short Button,short Shift,long X,long Y)
{
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
EXGAUGELib::IGaugePtr var_Gauge = ((EXGAUGELib::IGaugePtr)(spGrid1->GetItems()->GetItemObject(spGrid1->GetItems()->GetItemByIndex(2))));
var_Gauge->PutTimerInterval(1000);
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutScrollBySingleLine(VARIANT_TRUE);
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
spGrid1->PutHasLines(EXGRIDLib::exThinLine);
spGrid1->PutScrollBySingleLine(VARIANT_TRUE);
spGrid1->GetColumns()->Add(L"Default");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Normal Item");
h = var_Items->AddItem("Gauge-Clock Inside");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->InsertControlItem(h,L"Exontrol.Gauge",vtMissing);
var_Items->PutItemHeight(h,256);
EXGAUGELib::IGaugePtr var_Gauge = ((EXGAUGELib::IGaugePtr)(var_Items->GetItemObject(h)));
var_Gauge->PutPicturesPath(L"C:\\Program Files\\Exontrol\\ExGauge\\Sample\\Design\\Circular\\Clock");
var_Gauge->PutDefaultLayer(EXGAUGELib::exDefLayerRotateType,long(2));
var_Gauge->GetLayers()->PutCount(4);
EXGAUGELib::ILayerPtr var_Layer = var_Gauge->GetLayers()->GetItem(long(0));
var_Layer->GetBackground()->GetPicture()->PutName("vista_clock.png");
EXGAUGELib::ILayerPtr var_Layer1 = var_Gauge->GetLayers()->GetItem(long(1));
var_Layer1->PutPosition(3);
var_Layer1->PutKey("sec");
var_Layer1->PutOnDrag(EXGAUGELib::exDoRotate);
var_Layer1->PutSelectable(VARIANT_FALSE);
var_Layer1->GetBackground()->GetPicture()->PutName("second-hand.png");
var_Layer1->PutValueToRotateAngle(_bstr_t("((2:=(((1:=( ( (0:=(value < 0 ? floor(value) + 1 - value : value - floor(value))) < 0.5 ? =:0 : (0:= (=:0 - 0.5)) ) * 24 )) - ") +
"floor(=:1)) * 60 )) - floor(=:2) ) * 360");
var_Layer1->PutRotateAngleToValue(L"value / 360 / 24 / 60");
EXGAUGELib::ILayerPtr var_Layer2 = var_Gauge->GetLayers()->GetItem(long(2));
var_Layer2->PutPosition(2);
var_Layer2->PutKey("min");
var_Layer2->PutOnDrag(EXGAUGELib::exDoRotate);
var_Layer2->PutSelectable(VARIANT_FALSE);
var_Layer2->GetBackground()->GetPicture()->PutName("Minute.png");
var_Layer2->PutValueToRotateAngle(_bstr_t("((1:=( ( (0:=(value < 0 ? floor(value) + 1 - value : value - floor(value))) < 0.5 ? =:0 : (0:= (=:0 - 0.5)) ) * 24 )) - floor(") +
"=:1)) * 360");
var_Layer2->PutRotateAngleToValue(L"value / 360 / 24 / 60");
EXGAUGELib::ILayerPtr var_Layer3 = var_Gauge->GetLayers()->GetItem(long(3));
var_Layer3->PutPosition(1);
var_Layer3->PutKey("hour");
var_Layer3->PutOnDrag(EXGAUGELib::exDoRotate);
var_Layer3->GetBackground()->GetPicture()->PutName("Hour.png");
var_Layer3->PutValueToRotateAngle(L"2 * 360 * ( (0:=(value < 0 ? floor(value) + 1 - value : value - floor(value))) < 0.5 ? =:0 : (0:= (=:0 - 0.5)) )");
var_Layer3->PutRotateAngleToValue(L"value / 360 * 0.5");
_variant_t v = var_Gauge->FormatABC(L"date(`now`)",vtMissing,vtMissing,vtMissing);
var_Gauge->GetLayers()->GetItem("sec")->PutValue(v);
var_Gauge->GetLayers()->GetItem("min")->PutValue(v);
var_Gauge->GetLayers()->GetItem("hour")->PutValue(v);
h = var_Items->AddItem("Normal Item");
spGrid1->EndUpdate();
|
953
|
What's the difference between merge cells and divider item
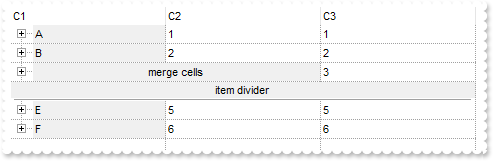
// AddItem event - Occurs after a new Item has been inserted to Items collection.
void OnAddItemGrid1(long Item)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellBackColor(Item,long(0),RGB(240,240,240));
var_Items->PutItemHasChildren(Item,VARIANT_TRUE);
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutTreeColumnIndex(0);
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
spGrid1->PutDrawGridLines(EXGRIDLib::exAllLines);
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"C1")))->PutFormatColumn(L"1 index `A-Z`");
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"C2")))->PutFormatColumn(L"1 index ``");
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"C3")))->PutFormatColumn(L"1 index ``");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem(vtMissing);
var_Items->AddItem(vtMissing);
long h = var_Items->AddItem(vtMissing);
var_Items->PutCellMerge(h,long(0),long(1));
var_Items->PutFormatCell(h,long(0),L"`merge cells`");
var_Items->PutCellHAlignment(h,long(0),EXGRIDLib::CenterAlignment);
h = var_Items->AddItem(vtMissing);
var_Items->PutItemDivider(h,0);
var_Items->PutCellHAlignment(h,long(0),EXGRIDLib::CenterAlignment);
var_Items->PutFormatCell(h,long(0),L"`item divider`");
var_Items->AddItem(vtMissing);
var_Items->AddItem(vtMissing);
spGrid1->EndUpdate();
|
952
|
is it possible to resize a column with the mouse without changing the width of the next column
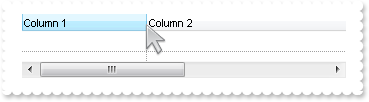
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Column 1")))->PutWidth(256);
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Column 2")))->PutWidth(512);
spGrid1->PutDrawGridLines(EXGRIDLib::exAllLines);
spGrid1->EndUpdate();
|
951
|
How do I ensure that the newly item fits the control's client area
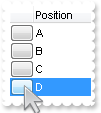
// ButtonClick event - Occurs when user clicks on the cell's button.
void OnButtonClickGrid1(long Item,long ColIndex,VARIANT Key)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("");
var_Items->PutSelectItem(h,VARIANT_TRUE);
var_Items->EnsureVisibleItem(h);
spGrid1->PutFocusColumnIndex(0);
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"")));
var_Column->PutAllowSizing(VARIANT_FALSE);
var_Column->PutAllowDragging(VARIANT_FALSE);
var_Column->PutAllowSort(VARIANT_FALSE);
var_Column->PutWidth(24);
var_Column->PutDef(EXGRIDLib::exCellHasButton,VARIANT_TRUE);
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Position")))->PutFormatColumn(L"1 apos `A-Z`");
spGrid1->PutCountLockedColumns(1);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("");
var_Items->AddItem("");
var_Items->AddItem("");
spGrid1->EndUpdate();
|
950
|
How do I find the predefined string for giving value, or giving identifier for specified predefined caption of editor
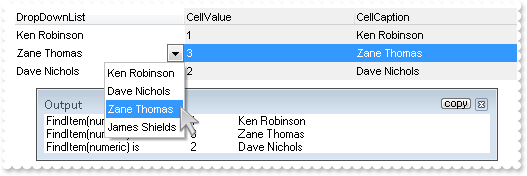
// Change event - Occurs when the user changes the cell's content.
void OnChangeGrid1(long Item,long ColIndex,VARIANT FAR* NewValue)
{
OutputDebugStringW( L"FindItem(numeric) is " );
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
OutputDebugStringW( L"NewValue" );
OutputDebugStringW( _bstr_t(spGrid1->GetColumns()->GetItem(long(0))->GetEditor()->GetFindItem(NewValue)) );
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"DropDownList")));
EXGRIDLib::IEditorPtr var_Editor = var_Column->GetEditor();
var_Editor->PutEditType(EXGRIDLib::DropDownListType);
var_Editor->AddItem(1,L"Ken Robinson",vtMissing);
var_Editor->AddItem(2,L"Dave Nichols",vtMissing);
var_Editor->AddItem(3,L"Zane Thomas",vtMissing);
var_Editor->AddItem(4,L"James Shields",vtMissing);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"CellValue")));
var_Column1->PutFormatColumn(L"%0");
var_Column1->PutDef(EXGRIDLib::exCellBackColor,long(15790320));
var_Column1->PutDef(EXGRIDLib::exHeaderBackColor,var_Column1->GetDef(EXGRIDLib::exCellBackColor));
EXGRIDLib::IColumnPtr var_Column2 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"CellCaption")));
var_Column2->PutFormatColumn(L"%C0");
var_Column2->PutDef(EXGRIDLib::exCellBackColor,long(15790320));
var_Column2->PutDef(EXGRIDLib::exHeaderBackColor,var_Column2->GetDef(EXGRIDLib::exCellBackColor));
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem(long(1));
var_Items->AddItem(spGrid1->GetColumns()->GetItem(long(0))->GetEditor()->GetFindItem("Zane Thomas"));
var_Items->AddItem(long(2));
spGrid1->EndUpdate();
|
949
|
How can I align captions of items with checkbox, with items with no checkbox

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->GetColumns()->Add(L"Default");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellImages(var_Items->AddItem(long(0)),long(0),"1");
var_Items->PutCellHasCheckBox(var_Items->AddItem(long(1)),long(0),VARIANT_TRUE);
var_Items->PutCellImages(var_Items->AddItem(long(2)),long(0),"1");
spGrid1->EndUpdate();
|
948
|
How can I prevent sorting a column
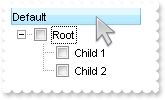
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Default")));
var_Column->PutDef(EXGRIDLib::exCellHasCheckBox,VARIANT_TRUE);
var_Column->PutPartialCheck(VARIANT_TRUE);
var_Column->PutAllowSort(VARIANT_FALSE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
spGrid1->EndUpdate();
|
947
|
Is there a possibility to group without moving and showing the column to the SortBar
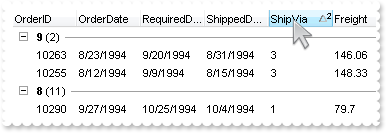
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutHasLines(EXGRIDLib::exNoLine);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutSingleSort(VARIANT_FALSE);
spGrid1->PutSortBarVisible(VARIANT_FALSE);
spGrid1->PutAllowGroupBy(VARIANT_TRUE);
spGrid1->PutLayout(L"singlesort=\"C5:1\";multiplesort=\" C1:2\"");
spGrid1->EndUpdate();
|
946
|
How can I show each group header ( not-subroup ), with a different background color, while alternate background colors for inside items
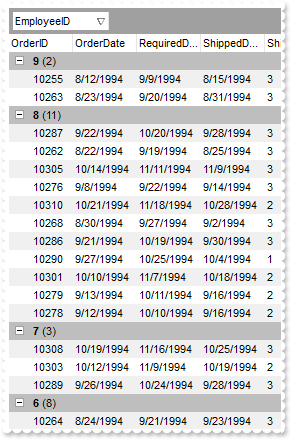
// LayoutChanged event - Occurs when column's position or column's size is changed.
void OnLayoutChangedGrid1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->Refresh();
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutHasLines(EXGRIDLib::exNoLine);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutSingleSort(VARIANT_FALSE);
spGrid1->PutSortBarVisible(VARIANT_TRUE);
spGrid1->PutAllowGroupBy(VARIANT_TRUE);
spGrid1->GetColumns()->GetItem(long(1))->PutSortOrder(VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Position")));
var_Column->PutFormatColumn(L"(0:= (1 rpos '')) right ( ( 1:= ( =:0 rfind `.` ) ) != -1 ? =:1 : len(=:0))");
var_Column->PutVisible(VARIANT_FALSE);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Position")));
var_Column1->PutFormatColumn(L"(1 rpos '') contains '.'");
var_Column1->PutVisible(VARIANT_FALSE);
spGrid1->GetConditionalFormats()->Add(L"(%C13 mod 2) != 0",vtMissing)->PutBackColor(RGB(240,240,240));
spGrid1->GetConditionalFormats()->Add(L"%C14 = 0",vtMissing)->PutBackColor(RGB(190,190,190));
spGrid1->EndUpdate();
|
945
|
What is the difference between %0 and %C0, when using in expressions ( format, conditional format, computed fields, and so on )
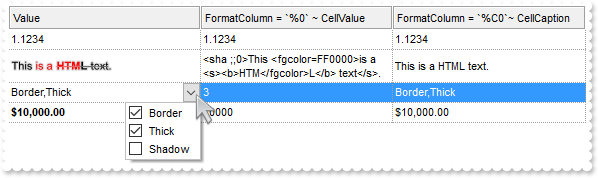
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutHeaderAppearance(EXGRIDLib::Etched);
spGrid1->PutHeaderHeight(24);
spGrid1->PutScrollBySingleLine(VARIANT_TRUE);
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Value")))->PutDef(EXGRIDLib::exCellValueFormat,long(1));
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"FormatColumn = `%0` ~ CellValue")));
var_Column->PutFormatColumn(L"%0");
var_Column->PutDef(EXGRIDLib::exCellSingleLine,VARIANT_FALSE);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"FormatColumn = `%C0`~ CellCaption")));
var_Column1->PutFormatColumn(L"%C0");
var_Column1->PutDef(EXGRIDLib::exCellSingleLine,VARIANT_FALSE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem(double(1.1234));
var_Items->PutCellValueFormat(var_Items->AddItem("<sha ;;0>This <fgcolor=FF0000>is a <s><b>HTM</fgcolor>L</b> text</s>."),long(0),EXGRIDLib::exHTML);
EXGRIDLib::IEditorPtr var_Editor = var_Items->GetCellEditor(var_Items->AddItem(long(3)),vtMissing);
var_Editor->PutEditType(EXGRIDLib::CheckListType);
var_Editor->AddItem(1,L"Border",vtMissing);
var_Editor->AddItem(2,L"Thick",vtMissing);
var_Editor->AddItem(4,L"Shadow",vtMissing);
var_Items->PutFormatCell(var_Items->AddItem(long(10000)),long(0),L"`<b>` + currency(value)");
spGrid1->EndUpdate();
|
944
|
How can I alternate colors for each group header ( not-subroup ), with a different background color, while items of the same group showing with a different color
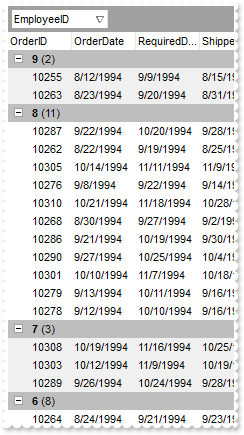
// LayoutChanged event - Occurs when column's position or column's size is changed.
void OnLayoutChangedGrid1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->Refresh();
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutHasLines(EXGRIDLib::exNoLine);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutSingleSort(VARIANT_FALSE);
spGrid1->PutSortBarVisible(VARIANT_TRUE);
spGrid1->PutAllowGroupBy(VARIANT_TRUE);
spGrid1->GetColumns()->GetItem(long(1))->PutSortOrder(VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Position")));
var_Column->PutFormatColumn(L"(0:= (1 rpos '')) left ( ( 1:= ( =:0 lfind `.` ) ) != -1 ? =:1 : len(=:0))");
var_Column->PutVisible(VARIANT_FALSE);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Position")));
var_Column1->PutFormatColumn(L"(1 rpos '') contains '.'");
var_Column1->PutVisible(VARIANT_FALSE);
spGrid1->GetConditionalFormats()->Add(L"(%C13 mod 2) != 0",vtMissing)->PutBackColor(RGB(240,240,240));
spGrid1->GetConditionalFormats()->Add(L"%C14 = 0",vtMissing)->PutBackColor(RGB(190,190,190));
spGrid1->EndUpdate();
|
943
|
How can I highlight each group header, with a different background color (method 2)
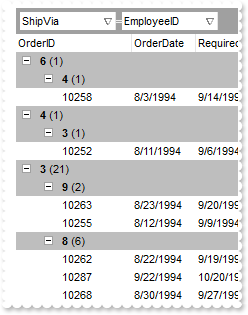
// AddGroupItem event - Occurs after a new Group Item has been inserted to Items collection.
void OnAddGroupItemGrid1(long Item)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->GetItems()->PutItemBackColor(Item,RGB(190,190,190));
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutHasLines(EXGRIDLib::exNoLine);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutSingleSort(VARIANT_FALSE);
spGrid1->PutSortBarVisible(VARIANT_TRUE);
spGrid1->PutAllowGroupBy(VARIANT_TRUE);
spGrid1->GetColumns()->GetItem(long(1))->PutSortOrder(VARIANT_TRUE);
spGrid1->EndUpdate();
|
942
|
How can I highlight each group header ( not-subroup ), with a different background color (method 1)
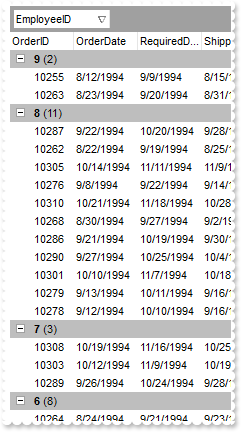
// LayoutChanged event - Occurs when column's position or column's size is changed.
void OnLayoutChangedGrid1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->Refresh();
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutHasLines(EXGRIDLib::exNoLine);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutSingleSort(VARIANT_FALSE);
spGrid1->PutSortBarVisible(VARIANT_TRUE);
spGrid1->PutAllowGroupBy(VARIANT_TRUE);
spGrid1->GetColumns()->GetItem(long(1))->PutSortOrder(VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Position")));
var_Column->PutFormatColumn(L"(0:= (1 rpos '')) left ( ( 1:= ( =:0 lfind `.` ) ) != -1 ? =:1 : len(=:0))");
var_Column->PutVisible(VARIANT_FALSE);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Position")));
var_Column1->PutFormatColumn(L"(1 rpos '') contains '.'");
var_Column1->PutVisible(VARIANT_FALSE);
spGrid1->GetConditionalFormats()->Add(L"%C14 = 0",vtMissing)->PutBackColor(RGB(190,190,190));
spGrid1->EndUpdate();
|
941
|
The BackColorAlternate displays each second row with a different background color. Is it possible to apply a different background color, for each sub-tree, ConditionalFormats, Add
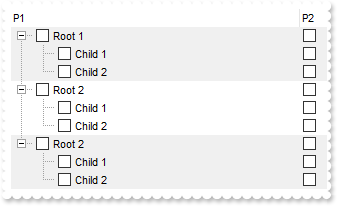
// LayoutChanged event - Occurs when column's position or column's size is changed.
void OnLayoutChangedGrid1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->Refresh();
}
// Sort event - Fired when the control sorts a column.
void OnSortGrid1()
{
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->Refresh();
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"P1")));
var_Column->PutDef(EXGRIDLib::exCellHasCheckBox,VARIANT_TRUE);
var_Column->PutPartialCheck(VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"P2")));
var_Column1->PutDef(EXGRIDLib::exCellHasCheckBox,VARIANT_TRUE);
var_Column1->PutPartialCheck(VARIANT_TRUE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column2 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Position")));
var_Column2->PutFormatColumn(L"(0:= (1 rpos '')) left ( ( 1:= ( =:0 lfind `.` ) ) != -1 ? =:1 : len(=:0))");
var_Column2->PutVisible(VARIANT_FALSE);
spGrid1->GetConditionalFormats()->Add(L"(%C2 mod 2) != 0",vtMissing)->PutBackColor(RGB(240,240,240));
spGrid1->EndUpdate();
|
940
|
The BackColorAlternate displays each second row with a different background color. Is it possible to apply a different background color, for 2nd, 3rd, 4th, row, and so on
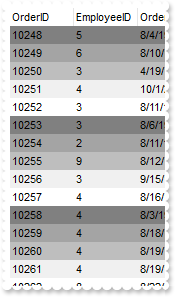
// LayoutChanged event - Occurs when column's position or column's size is changed.
void OnLayoutChangedGrid1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->Refresh();
}
// Sort event - Fired when the control sorts a column.
void OnSortGrid1()
{
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->Refresh();
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutHasLines(EXGRIDLib::exNoLine);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Position")));
var_Column->PutFormatColumn(L"1 apos ''");
var_Column->PutVisible(VARIANT_FALSE);
spGrid1->GetConditionalFormats()->Add(L"(%C13 mod 5) = 1",vtMissing)->PutBackColor(RGB(128,128,128));
spGrid1->GetConditionalFormats()->Add(L"(%C13 mod 5) = 2",vtMissing)->PutBackColor(RGB(164,164,164));
spGrid1->GetConditionalFormats()->Add(L"(%C13 mod 5) = 3",vtMissing)->PutBackColor(RGB(190,190,190));
spGrid1->GetConditionalFormats()->Add(L"(%C13 mod 5) = 4",vtMissing)->PutBackColor(RGB(240,240,240));
spGrid1->EndUpdate();
|
939
|
The BackColorAlternate displays each second row with a different background color. The question I have it is possible to apply a different background color for 3rd, 4th, row, and so on
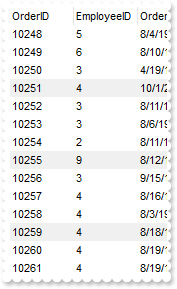
// LayoutChanged event - Occurs when column's position or column's size is changed.
void OnLayoutChangedGrid1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->Refresh();
}
// Sort event - Fired when the control sorts a column.
void OnSortGrid1()
{
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->Refresh();
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutHasLines(EXGRIDLib::exNoLine);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Position")));
var_Column->PutFormatColumn(L"1 apos ''");
var_Column->PutVisible(VARIANT_FALSE);
spGrid1->GetConditionalFormats()->Add(L"(%C13 mod 4) = 0",vtMissing)->PutBackColor(RGB(240,240,240));
spGrid1->EndUpdate();
|
938
|
The BackColorAlternate looks fine for flat tables, but how about using it when displaying a hierarchy/tree, like grouping rows. The sample alternate colors for each group found
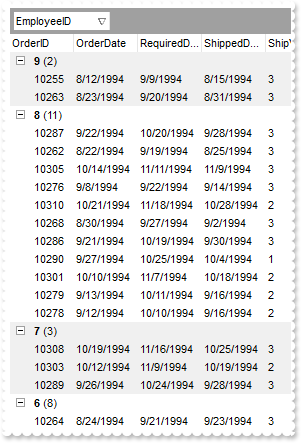
// LayoutChanged event - Occurs when column's position or column's size is changed.
void OnLayoutChangedGrid1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->Refresh();
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutHasLines(EXGRIDLib::exNoLine);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutSingleSort(VARIANT_FALSE);
spGrid1->PutSortBarVisible(VARIANT_TRUE);
spGrid1->PutAllowGroupBy(VARIANT_TRUE);
spGrid1->GetColumns()->GetItem(long(1))->PutSortOrder(VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Position")));
var_Column->PutFormatColumn(L"(0:= (1 rpos '')) left ( ( 1:= ( =:0 lfind `.` ) ) != -1 ? =:1 : len(=:0))");
var_Column->PutVisible(VARIANT_FALSE);
spGrid1->GetConditionalFormats()->Add(L"(%C13 mod 2) != 0",vtMissing)->PutBackColor(RGB(240,240,240));
spGrid1->EndUpdate();
|
937
|
I need to display sub-totals in the grouping items. Is there any solution on this
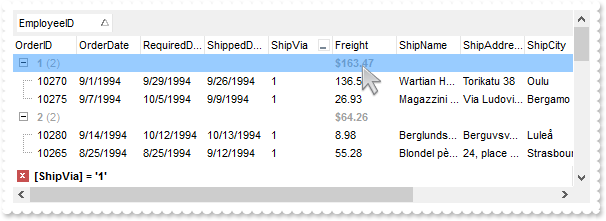
// AddGroupItem event - Occurs after a new Group Item has been inserted to Items collection.
void OnAddGroupItemGrid1(long Item)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutItemDivider(Item,-1);
var_Items->PutEnableItem(Item,VARIANT_FALSE);
var_Items->PutCellValueFormat(Item,spGrid1->GetTreeColumnIndex(),EXGRIDLib::exHTML);
var_Items->PutFormatCell(Item,spGrid1->GetTreeColumnIndex(),L"%1");
var_Items->PutCellValueFormat(Item,"Freight",EXGRIDLib::ValueFormatEnum(EXGRIDLib::exTotalField | EXGRIDLib::exHTML));
var_Items->PutCellValue(Item,"Freight","sum(current,dir,%6)");
var_Items->PutFormatCell(Item,"Freight",L"`<b>` + currency(value)");
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutSelBackMode(EXGRIDLib::exTransparent);
spGrid1->PutBackColorSortBar(RGB(240,240,240));
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutSortBarVisible(VARIANT_TRUE);
spGrid1->PutSortBarCaption(L"Drag a <b>column</b> header here to group by that column.");
spGrid1->PutAllowGroupBy(VARIANT_TRUE);
spGrid1->GetColumns()->GetItem(long(1))->PutSortOrder(EXGRIDLib::SortAscending);
spGrid1->PutLinesAtRoot(EXGRIDLib::exGroupLinesOutside);
spGrid1->GetColumns()->GetItem("ShipVia")->PutDisplayFilterButton(VARIANT_TRUE);
spGrid1->EndUpdate();
|
936
|
I use a subtotal in exTop-Item, after grouping the item shows 0. What is the solution
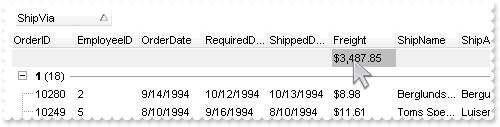
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutSingleSort(VARIANT_FALSE);
spGrid1->PutAllowGroupBy(VARIANT_TRUE);
spGrid1->PutSortBarVisible(VARIANT_TRUE);
spGrid1->PutBackColorSortBar(spGrid1->GetBackColor());
spGrid1->GetColumns()->GetItem(long(5))->PutSortOrder(EXGRIDLib::SortAscending);
spGrid1->GetColumns()->GetItem(long(6))->PutFormatColumn(L"currency(value)");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutLockedItemCount(EXGRIDLib::exTop,1);
long h = var_Items->GetLockedItem(EXGRIDLib::exTop,0);
var_Items->PutItemBackColor(h,RGB(240,240,240));
var_Items->PutCellBackColor(h,long(6),RGB(190,190,190));
var_Items->PutCellValue(h,long(6),"sum(all,rec,%6)");
var_Items->PutCellValueFormat(h,long(6),EXGRIDLib::exTotalField);
spGrid1->Refresh();
spGrid1->EndUpdate();
|
935
|
I would like to avoid manual typing in the date-cell because user often type wrong things (no decimal points and so on) and so the todays-date is generated for the cell. What can be done
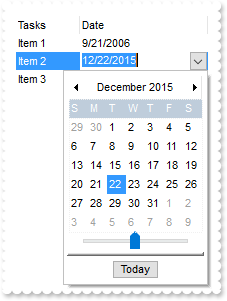
// KeyPress event - Occurs when the user presses and releases an ANSI key.
void OnKeyPressGrid1(short FAR* KeyAscii)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
OutputDebugStringW( L"if .Editying != 0 then" );
OutputDebugStringW( _bstr_t(spGrid1->GetEditing()) );
KeyAscii = 0;
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
var_Columns->Add(L"Tasks");
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Date")));
var_Column->GetEditor()->PutEditType(EXGRIDLib::DateType);
var_Column->PutWidth(128);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(var_Items->AddItem("Item 1"),long(1),COleDateTime(2006,9,21,0,00,00).operator DATE());
var_Items->PutCellValue(var_Items->AddItem("Item 2"),long(1),COleDateTime(2015,12,22,0,00,00).operator DATE());
var_Items->PutCellValue(var_Items->AddItem("Item 3"),long(1),COleDateTime(2015,1,10,0,00,00).operator DATE());
spGrid1->EndUpdate();
|
934
|
The control does not ensure the item to fit the control's client area once the user clicks the cell's button or check box. What can be done
// MouseDown event - Occurs when the user presses a mouse button.
void OnMouseDownGrid1(short Button,short Shift,long X,long Y)
{
// Items.EnsureVisibleItem(ItemFromPoint(-1,-1,c,hit))
}
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutTreeColumnIndex(-1);
spGrid1->PutSelForeColor(spGrid1->GetForeColor());
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Buttons")));
var_Column->PutAlignment(EXGRIDLib::CenterAlignment);
var_Column->PutDef(EXGRIDLib::exCellHasButton,VARIANT_TRUE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("Button A");
var_Items->AddItem("Button B");
var_Items->AddItem("Button C");
spGrid1->EndUpdate();
|
933
|
How do you save the index number from a drop down to a database
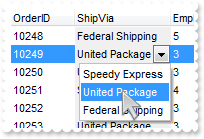
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
EXGRIDLib::IColumnPtr var_Column = spGrid1->GetColumns()->GetItem("ShipVia");
var_Column->PutDef(EXGRIDLib::exCellBackColor,long(15790320));
var_Column->PutPosition(1);
var_Column->PutWidth(96);
EXGRIDLib::IEditorPtr var_Editor = var_Column->GetEditor();
var_Editor->PutEditType(EXGRIDLib::DropDownListType);
var_Editor->AddItem(1,L"Speedy Express",vtMissing);
var_Editor->AddItem(2,L"United Package",vtMissing);
var_Editor->AddItem(3,L"Federal Shipping",vtMissing);
spGrid1->EndUpdate();
|
932
|
Is there a way to set the column width and have it stay when refreshing using the data source
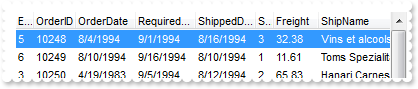
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutLayout(_bstr_t("gBjAAwAAuABmABpABsAB0ABlAByhoAPIAOEPAA9gYABoABQAgUEg0XN4AOcJicKkpujIAGMcj0gjcGk8QhkQgUOjUEjsfkMFAB2lEnhRihcYjUvnsykQAO8oMkTNEtG") +
"gAGUwn0uoEIhUMh0QiUOisXiE7rEyl8jAElokptYAllmpcCtMmjE3mU6jdzrUGoUKttGvFJs90oFPhVRh1Twg4wtaptco9fiMTsdIvcxw1Nkl2hUOlVwlsvnmayFAmtH" +
"nETuWm0lAv+eAGCzFK10zp1QqVUAGOvkvuuSr0YsMUi2Y0tZ4FAztt0FvuNa23Kvt2m0YnMt5No6uxwOq0eP5cGxAAxQAxgAGwAqu/q1blHDsGW49lzPUq9qtko58r8K" +
"rvc/LrPA7LWvw2ChpQ2j7Om7kBPK870hu+6ZQE4SJvmsT6u0x8BOa/iUP8jUANNATUrxAsKIFATvQU8DCL5B7dMWlr1u2gQZvgrsMMrDSyQ4vkcv02T+tEjUcyC1C7uw" +
"1kVABISgwSosXq1JLyRm9EaycqqDQuyjisu+0bvY5i3udEMjTIvkuQHFEmzHNkWymwcqtNNkIN2jUbMeGsdMm4j6R/AyZT7IcztC6M+r5Qs2yYvUx0ZOTZypBqBUZPEa" +
"Max71y6+MeTBDdBoFTjjv2z80Oi9a+VJE9HSA01SUlBbw1Ww8sPSqtFNNCdOx3L9AuQx9eVLIlUJbCa+WHVrV0fYUEMBF06UqAFh0xLLCT7abHV7P8MuNQUnW3YlDugl" +
"rfNNcVlrzV6s3FWVKMfcVrPSq6rva0wcz8+Ue2/YK+XzQ0QUQlt83/JTr2ZdiZYBKNoTm2rH4ZedzSckqGDqAA2tEk7CSQmKEJKgWMY5CmQJlkaZYsAGMY0liFJYqKID" +
"xhSpjCu0vUBQappOgSJZUhlBtTmyJIEqaBZVljRZe8yMZnoMl4SjQRI3qal3U7OjqPpOXNFmIAadCiHIkiDV6RjOla7puFKFnjK5/ta7Z6t6GIYkqEIQ1NmqWgIA=");
spGrid1->EndUpdate();
|
931
|
Is it possible to decode/view the control's Layout property
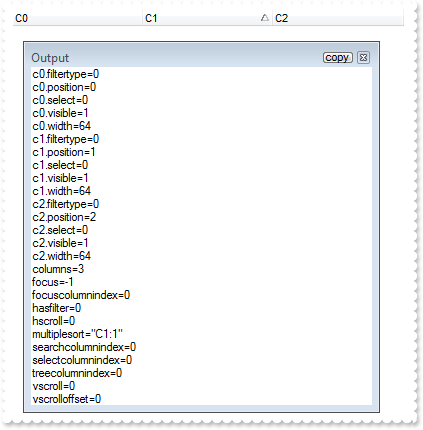
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutSingleSort(VARIANT_FALSE);
spGrid1->GetColumns()->Add(L"C0");
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"C1")))->PutSortOrder(EXGRIDLib::SortAscending);
spGrid1->GetColumns()->Add(L"C2");
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXPRINTLib' for the library: 'ExPrint 1.0 Control Library'
#import <ExPrint.dll>
using namespace EXPRINTLib;
*/
EXPRINTLib::IExPrintPtr var_Print = ::CreateObject(L"Exontrol.Print");
OutputDebugStringW( var_Print->GetDecode64TextW(spGrid1->GetLayout()) );
spGrid1->EndUpdate();
|
930
|
How do I programmatically sort by multiple columns

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutSingleSort(VARIANT_FALSE);
spGrid1->GetColumns()->Add(L"C0");
spGrid1->GetColumns()->Add(L"C1");
spGrid1->GetColumns()->Add(L"C2");
spGrid1->PutLayout(L"multiplesort=\"C2:1 C1:2 C0:2\"");
spGrid1->EndUpdate();
|
929
|
Do you have any Fit-To-Page options when printing the control (W x T, Fit-To )
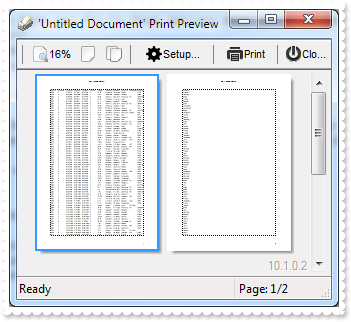
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
spGrid1->PutContinueColumnScroll(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->EndUpdate();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXPRINTLib' for the library: 'ExPrint 1.0 Control Library'
#import <ExPrint.dll>
using namespace EXPRINTLib;
*/
EXPRINTLib::IExPrintPtr var_Print = ::CreateObject(L"Exontrol.Print");
var_Print->PutOptions("FitToPage =2 x 1");
var_Print->PutPrintExt(((EXGRIDLib::IGridPtr)(spGrid1)));
var_Print->Preview();
|
928
|
Do you have any Fit-To-Page options when printing the control ( x T, Fit-To Tall )
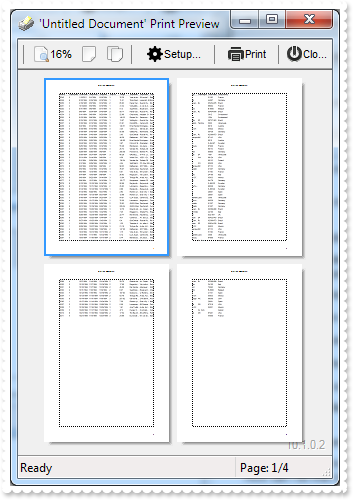
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
spGrid1->PutContinueColumnScroll(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->EndUpdate();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXPRINTLib' for the library: 'ExPrint 1.0 Control Library'
#import <ExPrint.dll>
using namespace EXPRINTLib;
*/
EXPRINTLib::IExPrintPtr var_Print = ::CreateObject(L"Exontrol.Print");
var_Print->PutOptions("FitToPage = x 2");
var_Print->PutPrintExt(((EXGRIDLib::IGridPtr)(spGrid1)));
var_Print->Preview();
|
927
|
Do you have any Fit-To-Page options when printing the control ( W x, Fit-To Wide )
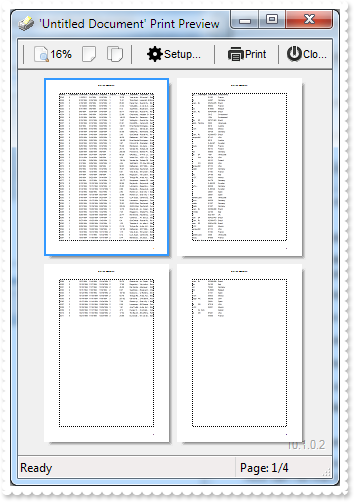
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
spGrid1->PutContinueColumnScroll(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->EndUpdate();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXPRINTLib' for the library: 'ExPrint 1.0 Control Library'
#import <ExPrint.dll>
using namespace EXPRINTLib;
*/
EXPRINTLib::IExPrintPtr var_Print = ::CreateObject(L"Exontrol.Print");
var_Print->PutOptions("FitToPage = 2 x");
var_Print->PutPrintExt(((EXGRIDLib::IGridPtr)(spGrid1)));
var_Print->Preview();
|
926
|
Do you have any Fit-To-Page options when printing the control ( percent view, Adjust-To )
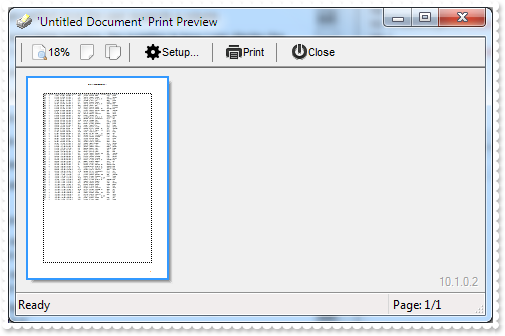
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
spGrid1->PutContinueColumnScroll(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->EndUpdate();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXPRINTLib' for the library: 'ExPrint 1.0 Control Library'
#import <ExPrint.dll>
using namespace EXPRINTLib;
*/
EXPRINTLib::IExPrintPtr var_Print = ::CreateObject(L"Exontrol.Print");
var_Print->PutOptions("FitToPage = 50%");
var_Print->PutPrintExt(((EXGRIDLib::IGridPtr)(spGrid1)));
var_Print->Preview();
|
925
|
How can I get notified once the user expands a column
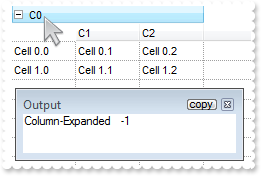
// LayoutChanged event - Occurs when column's position or column's size is changed.
void OnLayoutChangedGrid1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
OutputDebugStringW( L"Column-Expanded" );
OutputDebugStringW( _bstr_t(spGrid1->GetColumns()->GetItem("C0")->GetExpanded()) );
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutShowFocusRect(VARIANT_FALSE);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
spGrid1->PutDrawGridLines(EXGRIDLib::exAllLines);
spGrid1->PutBackColorLevelHeader(spGrid1->GetBackColor());
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C0")));
var_Column->PutExpandColumns(L"0,1,2");
var_Column->PutDisplayExpandButton(VARIANT_TRUE);
var_Columns->Add(L"C1");
var_Columns->Add(L"C2");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Cell 0.0");
var_Items->PutCellValue(h,long(1),"Cell 0.1");
var_Items->PutCellValue(h,long(2),"Cell 0.2");
h = var_Items->AddItem("Cell 1.0");
var_Items->PutCellValue(h,long(1),"Cell 1.1");
var_Items->PutCellValue(h,long(2),"Cell 1.2");
spGrid1->EndUpdate();
|
924
|
I am using expandable headers, the question is how I can display the column itself, not just the child columns
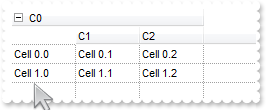
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
spGrid1->PutDrawGridLines(EXGRIDLib::exAllLines);
spGrid1->PutBackColorLevelHeader(spGrid1->GetBackColor());
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C0")));
var_Column->PutExpandColumns(L"0,1,2");
var_Column->PutDisplayExpandButton(VARIANT_TRUE);
var_Columns->Add(L"C1");
var_Columns->Add(L"C2");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Cell 0.0");
var_Items->PutCellValue(h,long(1),"Cell 0.1");
var_Items->PutCellValue(h,long(2),"Cell 0.2");
h = var_Items->AddItem("Cell 1.0");
var_Items->PutCellValue(h,long(1),"Cell 1.1");
var_Items->PutCellValue(h,long(2),"Cell 1.2");
spGrid1->EndUpdate();
|
923
|
How do I layout expandable columns

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
spGrid1->PutDrawGridLines(EXGRIDLib::exAllLines);
spGrid1->PutBackColorLevelHeader(spGrid1->GetBackColor());
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C0")));
var_Column->PutExpandColumns(L"1,2");
var_Column->PutDisplayExpandButton(VARIANT_TRUE);
var_Columns->Add(L"C1");
var_Columns->Add(L"C2");
var_Columns->Add(L"C3");
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C4")));
var_Column1->PutExpandColumns(L"5,6");
var_Column1->PutDisplayExpandButton(VARIANT_TRUE);
var_Columns->Add(L"C5");
EXGRIDLib::IColumnPtr var_Column2 = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C6")));
var_Column2->PutExpandColumns(L"6,7");
var_Column2->PutDisplayExpandButton(VARIANT_TRUE);
var_Columns->Add(L"C7");
spGrid1->EndUpdate();
spGrid1->GetColumns()->GetItem("C4")->PutExpanded(VARIANT_FALSE);
|
922
|
How do I make the control read-only (method 2)
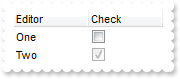
// Edit event - Occurs just before editing the focused cell.
void OnEditGrid1(long Item,long ColIndex,BOOL FAR* Cancel)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
Cancel = VARIANT_TRUE;
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IEditorPtr var_Editor = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Editor")))->GetEditor();
var_Editor->PutEditType(EXGRIDLib::CheckListType);
var_Editor->AddItem(1,L"One",vtMissing);
var_Editor->AddItem(2,L"Two",vtMissing);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Check")));
EXGRIDLib::IEditorPtr var_Editor1 = var_Column->GetEditor();
var_Editor1->PutEditType(EXGRIDLib::CheckValueType);
var_Editor1->PutOption(EXGRIDLib::exCheckValue1,long(2));
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(var_Items->AddItem(long(1)),long(1),long(0));
var_Items->PutCellValue(var_Items->AddItem(long(2)),long(1),long(1));
spGrid1->EndUpdate();
|
921
|
How do I set a locked check-box
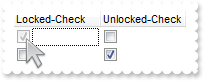
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Locked-Check")));
EXGRIDLib::IEditorPtr var_Editor = var_Column->GetEditor();
var_Editor->PutEditType(EXGRIDLib::CheckValueType);
var_Editor->PutOption(EXGRIDLib::exCheckValue1,long(2));
var_Editor->PutLocked(VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Unlocked-Check")));
EXGRIDLib::IEditorPtr var_Editor1 = var_Column1->GetEditor();
var_Editor1->PutEditType(EXGRIDLib::CheckValueType);
var_Editor1->PutOption(EXGRIDLib::exCheckValue2,long(1));
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(var_Items->AddItem(long(1)),long(1),long(0));
var_Items->PutCellValue(var_Items->AddItem(long(0)),long(1),long(1));
spGrid1->EndUpdate();
|
920
|
Does the title of the cell's tooltip supports HTML format
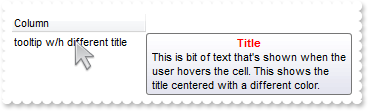
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"")));
var_Column->PutCaption(L"");
var_Column->PutHTMLCaption(L"Column");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellToolTip(var_Items->AddItem("tooltip w/h different title"),long(0),_bstr_t("<c><b><fgcolor=FF0000>Title</fgcolor></b><br>This is bit of text that's shown when the user hovers the cell. This shows the tit") +
"le centered with a different color.");
spGrid1->EndUpdate();
|
919
|
How do I specify a different title for the cell's tooltip
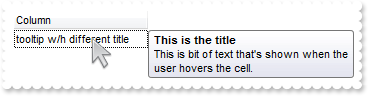
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"")));
var_Column->PutCaption(L"This is the title");
var_Column->PutHTMLCaption(L"Column");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellToolTip(var_Items->AddItem("tooltip w/h different title"),long(0),L"This is bit of text that's shown when the user hovers the cell.");
spGrid1->EndUpdate();
|
918
|
The cell's tooltip displays the column's caption in its title. How can I get ride of that
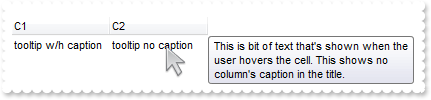
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
var_Columns->Add(L"C1");
var_Columns->Add(L"C2");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("tooltip w/h caption");
var_Items->PutCellToolTip(h,long(0),L"This is bit of text that's shown when the user hovers the cell. This shows the column's caption in the title.");
var_Items->PutCellValue(h,long(1),"tooltip no caption");
var_Items->PutCellToolTip(h,long(1),L"This is bit of text that's shown when the user hovers the cell. This shows no column's caption in the title.");
EXGRIDLib::IColumnPtr var_Column = spGrid1->GetColumns()->GetItem("C2");
var_Column->PutHTMLCaption(var_Column->GetCaption());
var_Column->PutCaption(L"");
spGrid1->EndUpdate();
|
917
|
How can I programmatically show the column's filter
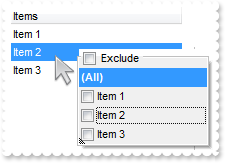
// RClick event - Fired when right mouse button is clicked
void OnRClickGrid1()
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
long i = spGrid1->GetItemFromPoint(-1,-1,c,hit);
spGrid1->GetColumns()->GetItem(c)->ShowFilter("-1,-1,128,128");
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutShowFocusRect(VARIANT_FALSE);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Items ")));
var_Column->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column->PutFilterList(EXGRIDLib::FilterListEnum(EXGRIDLib::exShowExclude | EXGRIDLib::exShowFocusItem | EXGRIDLib::exShowCheckBox));
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
var_Items->AddItem("Item 3");
spGrid1->EndUpdate();
|
916
|
I want to be able to click on one of the headers, and sort by other column. How can I do that (method 2)
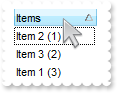
// ColumnClick event - Fired after the user clicks on column's header.
void OnColumnClickGrid1(LPDISPATCH Column)
{
// Column.SortOrder = 1
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->PutSortOnClick(EXGRIDLib::exDefaultSort);
spGrid1->GetColumns()->GetItem("Sort")->PutSortOrder(EXGRIDLib::SortAscending);
spGrid1->PutSortOnClick(EXGRIDLib::exUserSort);
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutSortOnClick(EXGRIDLib::exUserSort);
spGrid1->GetColumns()->Add(L"Items");
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Sort")))->PutVisible(VARIANT_FALSE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(var_Items->AddItem("Item 1 (3)"),long(1),long(3));
var_Items->PutCellValue(var_Items->AddItem("Item 2 (1)"),long(1),long(1));
var_Items->PutCellValue(var_Items->AddItem("Item 3 (2)"),long(1),long(2));
spGrid1->EndUpdate();
|
915
|
I want to be able to click on one of the headers, and sort by other column. How can I do that (method 1)
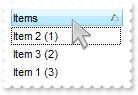
// ColumnClick event - Fired after the user clicks on column's header.
void OnColumnClickGrid1(LPDISPATCH Column)
{
// Column.SortOrder = 1
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->GetItems()->SortChildren(0,"Sort",VARIANT_TRUE);
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutSortOnClick(EXGRIDLib::exUserSort);
spGrid1->GetColumns()->Add(L"Items");
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Sort")))->PutVisible(VARIANT_FALSE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(var_Items->AddItem("Item 1 (3)"),long(1),long(3));
var_Items->PutCellValue(var_Items->AddItem("Item 2 (1)"),long(1),long(1));
var_Items->PutCellValue(var_Items->AddItem("Item 3 (2)"),long(1),long(2));
spGrid1->EndUpdate();
|
914
|
How can I highlight the cell's button with a different appearance, when cursor hovers it
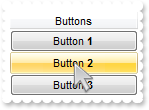
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spGrid1->PutDefaultItemHeight(22);
spGrid1->PutTreeColumnIndex(-1);
spGrid1->PutSelForeColor(RGB(0,0,0));
spGrid1->PutSelBackColor(spGrid1->GetBackColor());
spGrid1->PutBackground(EXGRIDLib::exCursorHoverCellButton,0x1000000);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Buttons")));
var_Column->PutDef(EXGRIDLib::exCellHasButton,VARIANT_TRUE);
var_Column->PutDef(EXGRIDLib::exCellValueFormat,long(1));
var_Column->PutAlignment(EXGRIDLib::CenterAlignment);
var_Column->PutHeaderAlignment(EXGRIDLib::CenterAlignment);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("Button <b>1</b>");
var_Items->AddItem("Button <b>2</b>");
var_Items->AddItem("Button <b>3</b>");
spGrid1->EndUpdate();
|
913
|
How can I prevent highlighting the cell's button while cursor hovers it
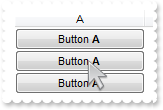
// AddItem event - Occurs after a new Item has been inserted to Items collection.
void OnAddItemGrid1(long Item)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(Item,long(0),"Button <b>A</b>");
var_Items->PutCellValue(Item,long(1),"Button <b>B</b>");
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutDefaultItemHeight(22);
spGrid1->PutTreeColumnIndex(-1);
spGrid1->PutSelForeColor(RGB(0,0,0));
spGrid1->PutSelBackColor(spGrid1->GetBackColor());
spGrid1->PutBackground(EXGRIDLib::exCursorHoverCellButton,-1);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"A")));
var_Column->PutDef(EXGRIDLib::exCellHasButton,VARIANT_TRUE);
var_Column->PutDef(EXGRIDLib::exCellValueFormat,long(1));
var_Column->PutAlignment(EXGRIDLib::CenterAlignment);
var_Column->PutHeaderAlignment(EXGRIDLib::CenterAlignment);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"B")));
var_Column1->PutDef(EXGRIDLib::exCellHasButton,VARIANT_TRUE);
var_Column1->PutDef(EXGRIDLib::exCellValueFormat,long(1));
var_Column1->PutAlignment(EXGRIDLib::CenterAlignment);
var_Column1->PutHeaderAlignment(EXGRIDLib::CenterAlignment);
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"")))->PutPosition(1);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellEnabled(var_Items->AddItem(""),long(1),VARIANT_FALSE);
var_Items->AddItem("");
var_Items->AddItem("");
spGrid1->EndUpdate();
|
912
|
How can I change the image of the icon while performing OLE Drag and Drop
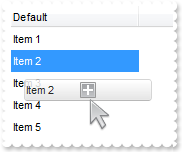
// OLEStartDrag event - Occurs when the OLEDrag method is called.
void OnOLEStartDragGrid1(LPDISPATCH Data,long FAR* AllowedEffects)
{
// Data.SetData("your data to drag")
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
AllowedEffects = 2;
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutOLEDropMode(EXGRIDLib::exOLEDropManual);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
spGrid1->PutDefaultItemHeight(22);
spGrid1->PutHeaderHeight(spGrid1->GetDefaultItemHeight());
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Default")))->PutWidth(128);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
var_Items->AddItem("Item 3");
var_Items->AddItem("Item 4");
var_Items->AddItem("Item 5");
EXGRIDLib::IAppearancePtr var_Appearance = spGrid1->GetVisualAppearance();
var_Appearance->Add(1,_bstr_t("gBFLBCJwBAEHhEJAAChABakMACAADACAxRDQNABQKAAzQFAYaBiG6GAAGEaRYgmFgAQhFcZQSKUOQTDKMIziYBYfgkMIgSbJUgDGAkRRdDSOYDmGQYDiCIoRShOMIjH") +
"LUXxtDaIZwhEAoJb+RgAUY/cTzaAEUwHHiTKInaCQIhsC4JUJAdRURQ9EwvCIZBpEWwLChENQwWLCNj2TScBwjCyqbale45ViqdoDU8lORLUi+M4zSBPcZVTRtGShPDB" +
"KTjMLaYgkIIlVpRNa0PC1GTzQ6mazkKQLRADDIDVbAeL3LiMBy9LyLLItQALByua5mWhbcZyBCOPgBTrRb5zO58FjuTK7YLjMB7NrUNYtFaUMy2OpOCADIaecTNcaWLx" +
"PF2MY1HWYxVj2Jw3DuRJonKYB5lKAYkkYdA6hyDIjBkApaFoAAhBMfYxiGNAkFECZnm4YQBgiOgDl2URSE4KAEj2AJKigFgGgGYIIAyPQ6CCRogAAOxhAMSgSDgIRDhY" +
"FoFmGCBmBQOAMjgdgQDsUITEIIg5iISAEmIOBigiJgqgqYhoFyVILyyMgyDmYxDg4rBjgiZg6g0Dh4kiTIMGMKAwmgOQkEkFhGhGZIJAoPoQAyQ4mE6BhlAkRgXhODoZ" +
"C0A4Pg6KRmCSFplkkdheDmJYTioVgACOY4uGaDwmgmJhqg8JlWmOGRmGkChyhyZxJAobYbmMI4yHqFQnkmdh+2RYp4DMIZ5gaBohmiCYGB+IJOmoNhtiPXZGG2I1tgyb" +
"4lmgGhmhqJt0Fyb4gk8CtsCiahKhYH4oXiAohiUKpKjaLt+goDJxiyaZqlaNot4OTJx3gKp2iiL5sAsBoov+KgMnOMZrisJpKjLjocnPeBLEaRI0m0SxWkSNBPEoDJ1j" +
"abJrHaXo35obOZC2OximaOZugsYpi3ga42mKO5vAuRpijsTxqAyd49m8S5mnqPuqFyd4gk+DAGn6QJwEwFp+kAT+BnmQpwgwNwOkPtYsnnrgsFcEpFnGDBnBKRRPiwUw" +
"ckecgMgcIpHGMKQwnuSZygyJ1/HKOgMnyS5zAyRwykycw5g4Eg0jCA57DqTpzkydw+kIDR9AUCY9A2HQXBIUh0g0JRJ5aUxmnQZIPnkUgvDUI5tFcVoPjUOB+A4QBAIC" +
"A");
var_Appearance->Add(2,_bstr_t("gBFLBCJwBAEHhEJAAChABC8MACAADACAxRDQNABQKAAzQFAYaBiG6GAAGEaRYgmFgAQhFcZQSKUOQTDKMIziaQIRDINQlSTJcQjKKEUQTFiXIyAKKwEgmEQMQiCcbzX") +
"IUBxAAqXZZFUaKAgOMJDTLBAzUTCQbR7HiQYyBeCQOo+VoaSACEIlAZJRjoOo5DJGGQILlQJqyYrpaAxIgkEJuTqGoQaXgle53PJeLpXW5Nez9P7AMBwK7bbaqeTyXa+" +
"eDtJhif4cXjIMhyLI8UxXEKOL7jDSYPgqK48QhCEJQPQ9EyXJqnahoemCeRXBZ+aqxbBsCwCep0YBeNr3HaNaz3PK/brtWxMDpeA5IYhhF7WdZFR4tMrOdAtHL9FyPJ5" +
"TFicgXnoTAKAsRpHPeVhrAUd4LkmY5yj+fQ+i8L4zk+Y5vjCe4oD6ZoNhSRxiisVRKg+T5vnWfB6h6J5yAIf4fieWJFHyHZHHSTAygyAociMKBKEKBIeCiCZyHYFAnCA" +
"B4mBeBQJlgRIegOCgYCySAgh2WAkgINAMmMNIgCcCYoGYLoLmKaIshqCgMliEICgmDRDEiUQmkmAhWDCD5inicIVg4TQYloJg2g2ExYhoJZJEidIThMCQSFyEwkGKaRK" +
"FEJQJBkOhLCUJIDFoRiKBmBJhDeDZZDoPAlgmQhghaGZimmHhphqZopDoYw3GYEgFgGHROGOFJkCSSQCDoTAkiSaQ6C6IBJFkPIUCSJ5CDoeQ5CcVZ5gWHROmONJsCMS" +
"ISByEFyjIRoYiaKYaG6HonEiOhcguJQIHoRJsh0WBWB2JIpiqShKi0OwqnqRouiyTpGhGBxiYIZKOhqGp2j4aRaAqZL3FAECAgA==");
spGrid1->PutBackground(EXGRIDLib::exDragDropBefore,0x1000000);
spGrid1->PutBackground(EXGRIDLib::exDragDropAfter,0x2000000);
spGrid1->PutBackground(EXGRIDLib::exDragDropForeColor,RGB(0,0,1));
spGrid1->EndUpdate();
|
911
|
How can I sort by two-columns, one by date and one by time
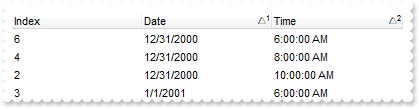
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutSingleSort(VARIANT_FALSE);
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Index")))->PutFormatColumn(L"1 index ``");
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Date")))->PutSortType(EXGRIDLib::SortDate);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Time")));
var_Column->PutSortType(EXGRIDLib::SortTime);
var_Column->PutFormatColumn(L"time(value)");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem(long(0));
var_Items->PutCellValue(h,long(1),COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Items->PutCellValue(h,long(2),COleDateTime(2001,1,1,10,00,00).operator DATE());
h = var_Items->AddItem(long(0));
var_Items->PutCellValue(h,long(1),COleDateTime(2000,12,31,0,00,00).operator DATE());
var_Items->PutCellValue(h,long(2),COleDateTime(2001,1,1,10,00,00).operator DATE());
h = var_Items->AddItem(long(0));
var_Items->PutCellValue(h,long(1),COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Items->PutCellValue(h,long(2),COleDateTime(2001,1,1,6,00,00).operator DATE());
h = var_Items->AddItem(long(0));
var_Items->PutCellValue(h,long(1),COleDateTime(2000,12,31,0,00,00).operator DATE());
var_Items->PutCellValue(h,long(2),COleDateTime(2001,1,1,8,00,00).operator DATE());
h = var_Items->AddItem(long(0));
var_Items->PutCellValue(h,long(1),COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Items->PutCellValue(h,long(2),COleDateTime(2001,1,1,8,00,00).operator DATE());
h = var_Items->AddItem(long(0));
var_Items->PutCellValue(h,long(1),COleDateTime(2000,12,31,0,00,00).operator DATE());
var_Items->PutCellValue(h,long(2),COleDateTime(2001,1,1,6,00,00).operator DATE());
spGrid1->PutLayout(L"multiplesort=\"C1:1 C2:1\"");
spGrid1->EndUpdate();
|
910
|
How can I display a context menu
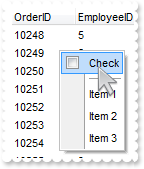
// MouseUp event - Occurs when the user releases a mouse button.
void OnMouseUpGrid1(short Button,short Shift,long X,long Y)
{
// Items.ToString = "Check[chk],[sep],Item 1,Item 2,Item 3"
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
ObjectPtr var_Object = ::CreateObject(L"Exontrol.ContextMenu");
;
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
spGrid1->PutScrollBySingleLine(VARIANT_TRUE);
spGrid1->PutContinueColumnScroll(VARIANT_FALSE);
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutReadOnly(EXGRIDLib::exReadOnly);
|
909
|
Also, are there any plans on the ability to put borders on individual cells or rows or columns
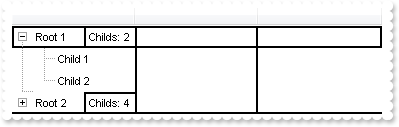
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IAppearancePtr var_Appearance = spGrid1->GetVisualAppearance();
var_Appearance->Add(1,_bstr_t("gBFLBCJwBAEHhEJAAChABOUGACAADACAxRDQNABQKAAzQFAYbBkGqGAAGIYxYgmFgAQhFcZQSpEEg7BKMYwjOJgFgmEQxDANIBQSKoaQiGQYRhkEYgEiONoaDJCM4wH") +
"IMQxHCKTZRkGYpajOPobUbGUywHRcRRvH6EZQGWg6GjqK43SCEEZhJBNGyTJ6BZbGURbCqSLAwWZAYy2RCMRxDJqLKypSwKPoGKosS5OUwzHItaRtHaJJAwKZ6ApGQpR" +
"VTAYxVfC1PzkACma4nS7oXraVJFVZTdYwTh+JABTzGLpnKw7FhGa5pABpdq0RTuOZdAbPMoyXBrXqqB46UCOGg5HRWWwHR7ZIquap9JzfCq5cRbWr5BBOPaBYKwdD1CB" +
"+iMVRnlQRY4hafZwAMH5Pl4XQnjCEBECSIBpDGHQOicIwtBIBpmiWEIJj6eJQloEgomafgyGGCI6kKYZQH+igGAKAJgEgFgGgGYIFlCf4CmCSA2A6A5hAgRgEgQYRIFY" +
"FIEmEaBmBmBghigdgQgcIZogYC4ICIKB6CSCRhiiHgogWIooi4F4AmKaIaDCDBihiTg0gsIIYmYOoOmOSJ2D6AZQBAgI=");
var_Appearance->Add(2,_bstr_t("gBFLBCJwBAEHhEJAAChABdUIQAAYAQGKIaBoAKBQAGaAoDDYMg1QwAAxDGLEEwsACEIrjKCVIgkHYJRjGEZxNCMIhiGAaQChEZYHgkMomDAOEgyHKcEgJGyEQgkOa4a") +
"jCKYrSzAcrwTI4cRVHiQZygOZ4DBSOY8VBAcQweItJhrKqVRgriitNQjCyjZCpOaIDooAJmRZNNISBBIEQSKA0TDOQ5TSKUMYhOZTBBEbbMNBtBIUIRpajbMBiFywUxU" +
"OJYXhmG4dR7IMhyLI8UxXFo7P7mOZZXjmO49T7Kc70LQ9CyHJKnabpWoaXj2VZZV7Mda2DTNSzPKK1bZpG4bTouKZ5WjfN72fgeCzrF7HchyPJcXxnG4ZAMBA");
var_Appearance->Add(3,_bstr_t("gBFLBCJwBAEHhEJAAChABL0GACAADACAxRDQNABQKAAzQFAYbBkGqGAAGIYxYgmFgAQhFcZQSpEEg7BKMYwjOJgEgmEQxDANIBQSKoaQiGQYRhkEYpFiONoXDJCM4wH") +
"IMQxHCKTZRkGYpajOPobUbGUygBRdExvEyEZQGWg6GjqK43SCEEZhJBNGyfH6dBpEWT7ChENQwWLLFoRDIcQyXCytIDter4boGKosS5OUwzGAtaRvHaJJAwKZ6ApGQpR" +
"VTAYxUdC1HTjJiEa4nS7oXraVJFVZTdYwTh+JABTzGKbsSycKqWaqkABZeoWbTuOZdAbPMoyXBrXgOLYzUCOGg5HRWWwHR7ZIq0Pg9Hqaa4bVbIVxbcAGH6BQa6J5hEB" +
"ECSIBpDGHQOicIwtBIBpmhqEIJj2eJQloEgokiegyGGCI6kKZ5BnefA+D8L4flOa52nufg+g+f5fnPFB/ooBZ1omSAWASAJgGgJgJgIIIoDYAIDCCaBFnuBAhCgOgUgU" +
"YIoF4GIBiGKBuAcfohmgNgdggX54g4JB/F+GImCqCpikiNguguUAQICA=");
var_Appearance->Add(4,_bstr_t("gBFLBCJwBAEHhEJAAChABBUGACAADACAxRDQNABQKAAzQFAYbBkGqGAAGIYxYgmFgAQhFcZQSpEEg7BKMYwjOJgCgmEQxDANMiwGKoaQiGQYRhkEYgFiONoaDJCM4wH") +
"IMQxHCKTZRkGYpajOPobUbAYQQSAkEgpECbZqoEZaDoaOorTZINJ0VR1Ox5KKfZyGURZPqOEQ1DBZEI2RZUbxDJquLhACj7AjeZZtRJZVp2TY9eQ3LC3aYhGqwAwSFpJ" +
"VjUEBgRBJIDSMY6DpOIxaEgNZpwEITOTxUK0EhRLy5agDCJ1QrCdanahqOpaXpmW5dV7YNh2LTnfzXNq3bhuO5bXqOd59X7fN54Dg+D4LRLHbpxXIcXqvFaZZDnOb4To" +
"PEuAZUmqcB2B2DoHGuN5Tm6d46lsPwfhOS5mnOeg9DqCAIICA");
spGrid1->PutLinesAtRoot(EXGRIDLib::exGroupLinesAtRoot);
spGrid1->PutSelBackColor(spGrid1->GetBackColor());
spGrid1->PutSelForeColor(spGrid1->GetForeColor());
spGrid1->PutDefaultItemHeight(22);
spGrid1->GetColumns()->Add(L"");
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"")))->PutDef(EXGRIDLib::exCellBackColor,long(33554432));
spGrid1->GetColumns()->Add(L"");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->PutItemBackColor(h,0x1000000);
_variant_t hx = var_Items->GetSplitCell(h,long(0));
var_Items->PutCellValue(long(0),hx,"count(current,dir,1)");
var_Items->PutCellValueFormat(long(0),hx,EXGRIDLib::exTotalField);
var_Items->PutFormatCell(long(0),hx,L"'Childs: ' + value");
var_Items->PutCellBackColor(long(0),hx,0x3000000);
var_Items->PutCellHAlignment(long(0),hx,EXGRIDLib::CenterAlignment);
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->PutItemBackColor(h,0x4000000);
hx = var_Items->GetSplitCell(h,long(0));
var_Items->PutCellValue(long(0),hx,"count(current,dir,1)");
var_Items->PutCellValueFormat(long(0),hx,EXGRIDLib::exTotalField);
var_Items->PutFormatCell(long(0),hx,L"'Childs: ' + value");
var_Items->PutCellBackColor(long(0),hx,0x3000000);
var_Items->PutCellHAlignment(long(0),hx,EXGRIDLib::CenterAlignment);
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->InsertItem(h,vtMissing,"Child 3");
var_Items->PutItemBackColor(var_Items->InsertItem(h,vtMissing,"Child 4"),0x4000000);
spGrid1->EndUpdate();
|
908
|
How can I decode the Layout property
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
var_Columns->Add(L"C1");
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"C2")))->PutPosition(1);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(var_Items->AddItem("SubItem 1.1"),long(1),"SubItem 1.2");
var_Items->PutCellValue(var_Items->AddItem("SubItem 2.1"),long(1),"SubItem 2.2");
spGrid1->GetColumns()->GetItem("C2")->PutSortOrder(EXGRIDLib::SortDescending);
spGrid1->EndUpdate();
OutputDebugStringW( L"Encoded:" );
OutputDebugStringW( spGrid1->GetLayout() );
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXPRINTLib' for the library: 'ExPrint 1.0 Control Library'
#import <ExPrint.dll>
using namespace EXPRINTLib;
*/
EXPRINTLib::IExPrintPtr var_Print = ::CreateObject(L"Exontrol.Print");
OutputDebugStringW( L"Decoded: " );
OutputDebugStringW( var_Print->GetDecode64TextW(spGrid1->GetLayout()) );
|
907
|
No new line is shown if using <br> tag. How can I show a new line with-in the cell
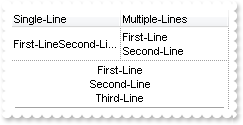
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutScrollBySingleLine(VARIANT_TRUE);
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Single-Line")));
var_Column->PutDef(EXGRIDLib::exCellSingleLine,VARIANT_TRUE);
var_Column->PutDef(EXGRIDLib::exCellValueFormat,long(1));
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Multiple-Lines")));
var_Column1->PutDef(EXGRIDLib::exCellSingleLine,VARIANT_FALSE);
var_Column1->PutDef(EXGRIDLib::exCellValueFormat,long(1));
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->PutCellValue(var_Items->AddItem("First-Line<br>Second-Line"),long(1),"First-Line<br>Second-Line");
long h = var_Items->AddItem("First-Line<br>Second-Line<br>Third-Line");
var_Items->PutCellSingleLine(h,vtMissing,EXGRIDLib::exCaptionWordWrap);
var_Items->PutCellHAlignment(h,long(0),EXGRIDLib::CenterAlignment);
var_Items->PutItemDivider(h,0);
spGrid1->EndUpdate();
|
906
|
I am using exCRD to layout the columns in the grid, but is there a way where I can have the text in a cell wrap if it's exceeds the width of the cell instead of showing the ...'s
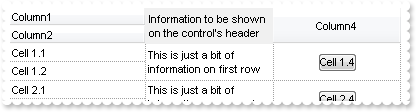
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutDrawGridLines(EXGRIDLib::exRowLines);
spGrid1->PutDefaultItemHeight(36);
spGrid1->PutFullRowSelect(EXGRIDLib::exColumnSel);
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Column1")));
var_Column->PutVisible(VARIANT_FALSE);
var_Column->GetEditor()->PutEditType(EXGRIDLib::EditType);
EXGRIDLib::IColumnPtr var_Column1 = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Column2")));
var_Column1->PutVisible(VARIANT_FALSE);
var_Column1->GetEditor()->PutEditType(EXGRIDLib::EditType);
EXGRIDLib::IColumnPtr var_Column2 = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Column3")));
var_Column2->PutVisible(VARIANT_FALSE);
var_Column2->PutDef(EXGRIDLib::exCellSingleLine,VARIANT_FALSE);
var_Column2->GetEditor()->PutEditType(EXGRIDLib::EditType);
EXGRIDLib::IColumnPtr var_Column3 = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Column4")));
var_Column3->PutAlignment(EXGRIDLib::CenterAlignment);
var_Column3->PutHeaderAlignment(EXGRIDLib::CenterAlignment);
var_Column3->PutVisible(VARIANT_FALSE);
var_Column3->PutDef(EXGRIDLib::exCellHasButton,VARIANT_TRUE);
var_Column3->PutDef(EXGRIDLib::exCellButtonAutoWidth,VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column4 = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"FormatLevel")));
var_Column4->PutFormatLevel(L"(0/1),\"Information to be shown on the control's header\"[a=17][ww]:128,3:128");
var_Column4->PutDef(EXGRIDLib::exCellFormatLevel,"(0/1),2[a=17][ww]:128,3:128");
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Cell 1.1");
var_Items->PutCellValue(h,long(1),"Cell 1.2");
var_Items->PutCellValue(h,long(2),"This is just a bit of information on first row");
var_Items->PutCellValue(h,long(3),"Cell 1.4");
var_Items->PutCellSingleLine(h,long(3),EXGRIDLib::exCaptionWordWrap);
h = var_Items->AddItem("Cell 2.1");
var_Items->PutCellValue(h,long(1),"Cell 2.2");
var_Items->PutCellValue(h,long(2),"This is just a bit of information on second row");
var_Items->PutCellValue(h,long(3),"Cell 2.4");
spGrid1->EndUpdate();
|
905
|
How can I load pictures using URL ( http:// )
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXHTTPLib' for the library: 'ExHTTP 1.0 Control Library'
#import <ExHTTP.dll>
using namespace EXHTTPLib;
*/
EXHTTPLib::IHTTPPtr http = ::CreateObject(L"Exontrol.HTTP");
spGrid1->PutPictureDisplay(EXGRIDLib::LowerRight);
spGrid1->PutPicture(IPictureDispPtr(((ObjectPtr)(http->GetGETImage(L"http://mail.exontrol.com/images/exontrol.png")))));
|
904
|
How can I filter programmatically by multiple columns
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
EXGRIDLib::IColumnsPtr var_Columns = spGrid1->GetColumns();
var_Columns->Add(L"Name");
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Active")));
var_Column->PutDef(EXGRIDLib::exCellHasCheckBox,VARIANT_TRUE);
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Columns->Add(L"Type");
((EXGRIDLib::IColumnPtr)(var_Columns->Add(L"Mode")))->PutFilterType(EXGRIDLib::exFilter);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long h = var_Items->AddItem("Item A");
var_Items->PutCellState(h,long(1),1);
var_Items->PutCellValue(h,long(2),"A");
h = var_Items->AddItem("Item B");
var_Items->PutCellState(h,long(1),0);
var_Items->PutCellValue(h,long(2),"B");
h = var_Items->AddItem("Item C");
var_Items->PutCellState(h,long(1),1);
var_Items->PutCellValue(h,long(2),"C");
var_Items->PutCellValue(h,long(3),"None");
h = var_Items->AddItem("Item D");
var_Items->PutCellState(h,long(1),1);
var_Items->PutCellValue(h,long(2),"C");
EXGRIDLib::IColumnPtr var_Column1 = spGrid1->GetColumns()->GetItem(long(1));
var_Column1->PutFilterType(EXGRIDLib::exCheck);
var_Column1->PutFilter(L"1");
EXGRIDLib::IColumnPtr var_Column2 = spGrid1->GetColumns()->GetItem(long(2));
var_Column2->PutFilterType(EXGRIDLib::exFilter);
var_Column2->PutFilter(L"C");
EXGRIDLib::IColumnPtr var_Column3 = spGrid1->GetColumns()->GetItem(long(3));
var_Column3->PutFilterType(EXGRIDLib::exNonBlanks);
spGrid1->ApplyFilter();
spGrid1->EndUpdate();
|
903
|
How can I add Right-To-Left Reading-Order / RTL Layout
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutTreeColumnIndex(-1);
EXGRIDLib::IColumnPtr var_Column = ((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"RTL - Header Caption")));
var_Column->PutHeaderAlignment(EXGRIDLib::AlignmentEnum(0x20000 | EXGRIDLib::RightAlignment));
var_Column->PutAlignment(EXGRIDLib::AlignmentEnum(0x20000 | EXGRIDLib::RightAlignment));
spGrid1->PutFullRowSelect(EXGRIDLib::exColumnSel);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("RTL - Text Right");
var_Items->PutCellHAlignment(var_Items->AddItem("RTL - Text Center"),long(0),EXGRIDLib::AlignmentEnum(0x20000 | EXGRIDLib::CenterAlignment));
var_Items->PutCellHAlignment(var_Items->AddItem("RTL - Text Left"),long(0),EXGRIDLib::AlignmentEnum(0x20000));
spGrid1->EndUpdate();
|
902
|
I have applied ebn to the grid using the following code, and noticed that it applies to the filter dropdownList too. Is there a way to prevent this behavior, like keeping the Filter dropdownlist intact
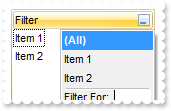
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spGrid1->PutAppearance(EXGRIDLib::AppearanceEnum(0x1000000));
spGrid1->PutBackColorHeader(0x1000000);
spGrid1->PutBackground(EXGRIDLib::exBackColorFilter,0x8000000f);
((EXGRIDLib::IColumnPtr)(spGrid1->GetColumns()->Add(L"Filter")))->PutDisplayFilterButton(VARIANT_TRUE);
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
var_Items->AddItem("Item 1");
var_Items->AddItem("Item 2");
|
901
|
The tree lines from the group parent to its children are missing and no identation is present: the parent and all its children are on the same offset from left. What canbe done
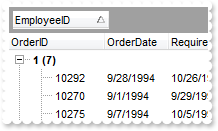
// AddGroupItem event - Occurs after a new Group Item has been inserted to Items collection.
void OnAddGroupItemGrid1(long Item)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGRIDLib' for the library: 'ExGrid 1.0 Control Library'
#import <ExGrid.dll>
using namespace EXGRIDLib;
*/
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
EXGRIDLib::IItemsPtr var_Items = spGrid1->GetItems();
long nGroupColumn = var_Items->GetGroupItem(Item);
var_Items->PutItemDivider(Item,-1);
var_Items->PutFormatCell(Item,long(0),var_Items->GetFormatCell(Item,nGroupColumn));
var_Items->PutCellValue(Item,long(0),spGrid1->GetColumns()->GetItem(nGroupColumn)->GetGroupByTotalField());
var_Items->PutCellValueFormat(Item,long(0),var_Items->GetCellValueFormat(Item,nGroupColumn));
}
EXGRIDLib::IGridPtr spGrid1 = GetDlgItem(IDC_GRID1)->GetControlUnknown();
spGrid1->BeginUpdate();
spGrid1->PutReadOnly(EXGRIDLib::exReadOnly);
spGrid1->PutColumnAutoResize(VARIANT_FALSE);
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rs = ::CreateObject(L"ADOR.Recordset");
rs->Open("Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExGrid\\Sample\\Access\\misc.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spGrid1->PutDataSource(((ADODB::_RecordsetPtr)(rs)));
spGrid1->PutSortBarVisible(VARIANT_TRUE);
spGrid1->PutSortBarCaption(L"Drag a <b>column</b> header here to group by that column.");
spGrid1->PutAllowGroupBy(VARIANT_TRUE);
EXGRIDLib::IColumnPtr var_Column = spGrid1->GetColumns()->GetItem(long(0));
var_Column->PutAllowGroupBy(VARIANT_FALSE);
var_Column->PutWidth(96);
spGrid1->GetColumns()->GetItem(long(1))->PutSortOrder(EXGRIDLib::SortAscending);
spGrid1->PutLinesAtRoot(EXGRIDLib::exLinesAtRoot);
spGrid1->EndUpdate();
|